Stack
Table of Contents [hide]
It is the child class of vector & contains only or constructor.
Stack s =new stack ()
Method of stack:-
- Object push ( object o ) // for inserting an object
- Object pop ( object o ) // remove &returns top of the stack
- Object Peek () // returns top of the stack with out removal
- Boolean empty () ; // return true, if stack is empty
- Int search ( object o )
If the element present, then it returns off set from the top of the stack, otherwise returns -1.
import java. util. * ; Class StackDemo { Stack s = new Stack (); { stack push ( "A" ); stack push ( "B" ); stack push ( "C" ); s.o.p (s); s.o.p ( stack search ( "A" ); // 3 s.o.p ( stack search ( "Z" ); // -1 } }
The cursor of java:-
To retrieve object by object from any collection object we should go to cursors. There are 3 types of cursors available in java.
- Enumeration.
- Iterator.
- Listiterator.
Enumeration
⇒ It is introduced in 1.0 version and it is legacy.
⇒ This interface define following 2 methods.
- Public boolean hasMoreElemnts ()
- Public object nextElement ()
⇒ We can get Enumeration object by using elements () method.
Enumeration e = vector elements () import java. util. * (); Class EnumerationDemo { public static void main( String[] args ) { vector v = new vector (); for ( i=0, i<=10, i++) { vector addElement ( i ); } s.o.p (v); // 0,1,2,........9,10 Enumeration e = vector elements (); while ( Enumeration has MoreElements ()) { integer I = ( Integer ) enumeration nextElement (); if ( I%2 = = 0) s.o.p (I); // 0,2,4,8,10 } s.o.p ( v ); // 0,1,2.........9,10 } }
The main limitation of Enumeration is while iterating we can’t perform removal operation iterator Enumeration just provide read access. we can resolve this problem by using iterator.An Enumeration is a group of named constants , it has introduced in 1.5 version.
Enumeration bear
{
KF, KO, RC, FO ;
}
Iterator
⇒ It has iterator from 1.2 version.
⇒ It is applicable for any collection objects, hence it is universal cursor.
⇒ iterator interface defines the following 3 methods
- boolean hasNext ()
- boolean next ()
- void remove ()
⇒ We can get iterator objector by using iterator of collection interface.
Iterator itr = collection iterator (); import java. util. * ; Class IteratorDemo { Public static void main ( String[] args ) { ArrayList l = new ArrayList (); for ( int i = 0; i<=10; i++ ) { l.add ( i ); } s.o.p ( l ); Iterator itr = l. iterator (); while ( itr. hasNext () ) { Iterger I = ( Integer ) IntergerNext () ) if ( I%2 = = 0 ) { s.o.p ( I ) // 0,2,4,6,8,10 } else { itr.remove (); } s.o.p ( l ); // 0, 2, 4, 6, 8 ,10 }
ListIterator
⇒ It is the child interface of iterator & it has introduced in 1.2 version.
⇒ By using listIterator, we can move either to the forward or to the backward direction i.e it is a bi directional cursor.
⇒ while iteratoring we can also perform addition of new objects, replaceing existing objects.
⇒We can get listIterator by using listIterator method.
ListIterator ltr = l.listIterator ();
Methods of ListIterator interface:-
- Boolean hasNext ();
- Boolean hasprevious ();
- Object next ();
- Object previous ();
- int nextIndex (); // If the next Element is not available, then this method return sizx of index.
- int PreviousIndex (); // If there is no previous Element, then this method return -1.
- Void remove ()
- Void set ( object new )
- Void add ( object new )
import java. util. * ; Class ListIteratorDemo { public static { LinkedList l = new LinkedList (); l.add ( "Narayana swamy"); l.add ( "Anitha"); l.add ( "Vighnesh"); l.add ( "Sunny"); s.o.p ( l ); ListIterator ltr = l.listIterator () ; while ( ltr. hasNext () ) { String s = ( String ) ltr. next () ; if ( s. equals ( "Narayana")) { ltr. remove () ; } } s.o.p ( l ); } } /* If ( s. equals ( " Narayana" ) { ltr. set ( " Anitha " ); } If (s. equals ( "Sunny" ); { ltr. set ( " Vighnesh " ); } */
Property |
Enumeration |
Iterator |
ListIteration |
Is it legacy | Yes | No | No |
Is it applicable for
|
Only legacy collection classes ( vector, stack) | Even collection object
|
Only list implemented class objects
|
Movements
|
Single directional cursor ( forward )
|
Single dimention (forward)
|
BiDirection
|
Accessability | Only read | Read & remove | Read, remove add, replace |
How to get | By elements () | By iterator () | By listIterator |
Method
|
2 methods hasMoreElements (), NextElement () | 3 methods hasNext (), remove () | A methods
|
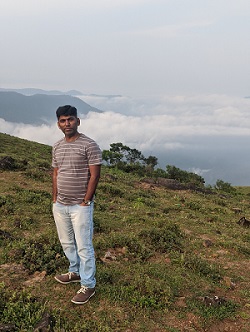
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.