Enhancement
Table of Contents
Sun people introduces, the following 2 interface in 1.6 version as the part of collection Framework.
1) NavigableSet
2) NavigableMap.
It is the child interface of SortedSet, which defines several methods to support navigation.
- Ceiling(e): It returns the lowest element, which is greater than equal to e.
- Higher(e): Returns the lowest element which is greater than ‘e’.
- Floor(e): Returns highest element which is less than or equal to e.
- Lower(e): Returns highest element which is less than.
- PollFirst(): Returns & removes the First element.
- PollLast(): Returns & removes the Last element.
- DescendingSet(): Returns the NavigableSet, in reverse order.
package com.narayanatutorial.collections.set;
import java.util.TreeSet;
public class NavigableSetDemo {
public static void main (String[] args)
{
TreeSet<Integer> t = new TreeSet<Integer> ();
t.add(1000);
t.add(2000);
t.add(3000);
t.add(4000);
t.add(5000);
System.out.println("treeset:"+t); // 1000,2000,3000,4000,5000
System.out.println("ceiling:"+t.ceiling(2000)); //2000
System.out.println("higher:"+t.higher(2000)); //3000
System.out.println("floor:"+t.floor(3000)); //3000
System.out.println("lower:"+t.lower(3000)); //2000
System.out.println("pollFirst:"+t.pollFirst()); //1000
System.out.println("pollLast:"+t.pollLast()); //5000
System.out.println("Final treeset:"+t); //2000,3000,4000
}
}
Output
treeset:[1000, 2000, 3000, 4000, 5000]
ceiling:2000
higher:3000
floor:3000
lower:2000
pollFirst:1000
pollLast:5000
Final treeset:[2000, 3000, 4000]
It is child interface of SortedMap. It defines several method to support Navigation.
Map⇒ SortedMap⇒ NavigableMap⇒ TreeMap.
- CeilingKey (e)
- HigherKey (e1)
- FloorKey (e1)
- LowerKey (e1)
- PollFirstEntry ()
- PollLastEntry()
- DescendingMap()
package com.narayanatutorial.collections.set;
import java.util.TreeMap;
public class NavigableMap {
public static void main(String[] args) {
// TODO Auto-generated method stub
TreeMap<String, String> t = new TreeMap<String, String>();
t.put("b", "banana");
t.put("a", "apple");
t.put("d", "dog");
t.put("c", "cat");
t.put("g", "gun");
System.out.println("TreeMap:"+t);
System.out.println("ceilingKey:"+t.ceilingKey("c"));
System.out.println("higherKey:"+t.higherKey("e"));
System.out.println("floorKey:"+t.floorKey("e"));
System.out.println("lowerKey:"+t.lowerKey("e"));
System.out.println("pollFirstEntry"+t.pollFirstEntry());
System.out.println("pollFirstEntry:"+t.pollLastEntry());
System.out.println("pollFirstEntry:"+t.descendingMap());
System.out.println("final TreeMap"+t);
}
}
Output
TreeMap:{a=apple, b=banana, c=cat, d=dog, g=gun}
ceilingKey:c
higherKey:g
floorKey:d
lowerKey:d
pollFirstEntrya=apple
pollFirstEntry:g=gun
pollFirstEntry:{d=dog, c=cat, b=banana}
final TreeMap{b=banana, c=cat, d=dog}
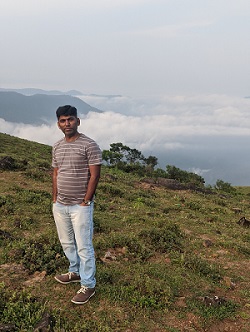
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.