Collections
Table of Contents
It is an utility class present in java.util package. It defines several utility methods for collection implemented class object.
Sorting a list
public static void sort(List l), to sort the elements of List, according to natural sorting order. In this case compulsory Objects should be Homogeneous & comparable otherwise we will get ClassCastException. The list should not null, otherwise NullPointerException.
Public static void sort(List l, Comparator c): To sort the elements of list according to customized sorting order described by comparative.
CollectionSort.java
package com.narayanatutorial.collections.list;
import java.util.ArrayList;
import java.util.Collections;
public class CollectionSort {
public static void main(String[] args) {
ArrayList<String> al = new ArrayList<String>();
al.add("Z");
al.add("B");
al.add("K");
al.add("N");
// al.add (new integer(10));// ClassCastException
// al.add(null);// NullPointerException
System.out.println("before sorting" + al);
Collections.sort(al);// [ZAKN]
System.out.println("after sorting" + al);// [AKNZ]
}
}
Output
before sorting[Z, B, K, N]
after sorting[B, K, N, Z]
MyComparator.java
package com.narayanatutorial.collections.list;
import java.util.Comparator;
public class MyComparator implements Comparator<Object>{
public int compare(Object Obj1, Object Obj2)
{
String s1 = (String)Obj1;
String s2 = (String)Obj2;
return s1.compareTo(s2);
}
}
CollectionsSortDemo.java
package com.narayanatutorial.collections.list;
import java.util.ArrayList;
import java.util.Collections;
public class CollectionsSortDemo {
public static void main (String[] args)
{
ArrayList<String> al= new ArrayList<String>();
al.add("Z");
al.add("B");
al.add("K");
al.add("L");
System.out.println("before sorting"+al);// [ZAKLL]
Collections.sort(al, new MyComparator ());
System.out.println("after sorting"+al);// [ZLLKA]
}
}
Output
before sorting[Z, B, K, L]
after sorting[B, K, L, Z]
Searching the list
Collections class defines the following binary search methods.
Public static int binarySearch(List l, Object O): We have to use this method, if list is sorted according to natural sorting order.
Public static in binarySearch(List l, Object O, Comparator c): We have to use this method, if the list is sorted according to comparator.
- Before calling binarySearch() method, compulsory the list should be sorted, otherwise we will get Unpredictable results.
- Successful search returns index, unsuccessful search returns insertion point. Insertion point is the location, where we can place the element.
- If the list is sorted, according to comparator then at the time of search operation also, we have to provide same comparator. Otherwise we will get Unpredictable result.
CollectionsSearchDemo.java
package com.narayanatutorial.collections.list;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class CollectionsSearchDemo {
public static void main(String[] args) {
List<String> al = new ArrayList<String>();
al.add("Z");
al.add("A");
al.add("M");
al.add("K");
al.add("a");
Collections.sort(al);
System.out.println("al:"+al);
System.out.println(Collections.binarySearch(al, "Z"));
System.out.println(Collections.binarySearch(al, "j"));
}
}
Output
al:[A, K, M, Z, a]
3
-6
MyComparatorInteger.java
package com.narayanatutorial.collections.list;
import java.util.Comparator;
public class MyComparatorInteger implements Comparator<Object> {
@Override
public int compare(Object o1, Object o2) {
// TODO Auto-generated method stub
Integer i1=(Integer)o1;
Integer i2=(Integer)o2;
return i1.compareTo(i2);
}
}
CollectionsSearchDemoComparator.java
package com.narayanatutorial.collections.list;
import java.util.ArrayList;
import java.util.Collections;
public class CollectionsSearchDemoComparator {
public static void main(String args[]) {
ArrayList<Integer> al = new ArrayList<Integer>();
al.add(15);
al.add(0);
al.add(20);
al.add(5);
Collections.sort(al, new MyComparatorInteger());
System.out.println("al:"+al);
System.out.println(Collections.binarySearch(al, 10, new MyComparatorInteger()));
System.out.println(Collections.binarySearch(al, 13, new MyComparatorInteger()));
System.out.println(Collections.binarySearch(al, 17));// unPredicatable
}
}
Output
al:[0, 5, 15, 20]
-3
-3
-4
Note
For the list, with ‘n’ elements, the possible values for successful binary search methods on 0 to n-1.
⇒ Unsuccessful search -1 to -(n+1).
⇒ total range -(n+1) to n-1.
Reversing the elements of a List
Collections class contain reverse() method public static void reverse(List l).
ExonReverse.java
package com.narayanatutorial.collections.list;
import java.util.ArrayList;
import java.util.Collections;
public class ExonReverse {
public static void main(String args[]) {
ArrayList<Integer> al = new ArrayList<Integer>();
al.add(15);
al.add(0);
al.add(20);
al.add(10);
al.add(5);
Collections.reverse(al);
System.out.println("Rerverse Order:"+al);
}
}
Output
Rerverse Order:[5, 10, 20, 0, 15]
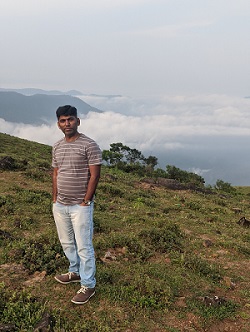
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.