How to send a mail using java Office365 SMTP server
Table of Contents
In this article, I am going to show How to send a mail using java Office365 SMTP server, Office365 SMTP server details and how to connect Office365 from java
Pre-Requisites
- java 1.8
- Eclipse
- Java Mail API
Office365 Connection Properties
String USERNAME = "[email protected]"; String PASSWORD = "XXXXXXX"; String HOSTNAME = "smtp.office365.com"; String STARTTLS_PORT = "587"; boolean STARTTLS = true; boolean AUTH = true; String FromAddress="[email protected]";
To Send Text Message Body Mail
mimeMessage.setText(EmailBody);
To Send HTML Message Body
mimeMessage.setContent(EmailBody,"text/html; charset=utf-8");
Send Mail
Transport.send(mimeMessage);
Office365TextMsgSend.java
package com.narayanatutorial.office365; import java.util.Properties; import javax.mail.Message.RecipientType; import javax.mail.Authenticator; import javax.mail.MessagingException; import javax.mail.PasswordAuthentication; import javax.mail.Session; import javax.mail.Transport; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeMessage; public class Office365TextMsgSend { Properties properties; Session session; MimeMessage mimeMessage; String USERNAME = "[email protected]"; String PASSWORD = "XXXXXXX"; String HOSTNAME = "smtp.office365.com"; String STARTTLS_PORT = "587"; boolean STARTTLS = true; boolean AUTH = true; String FromAddress="[email protected]"; public static void main(String args[]) throws MessagingException { String EmailSubject = "Subject:Text Subject"; String EmailBody = "Text Message Body: Hello World"; String ToAddress = "[email protected]"; Office365TextMsgSend office365TextMsgSend = new Office365TextMsgSend(); office365TextMsgSend.sendGmail(EmailSubject, EmailBody, ToAddress); } public void sendGmail(String EmailSubject, String EmailBody, String ToAddress) { try { properties = new Properties(); properties.put("mail.smtp.host", HOSTNAME); // Setting STARTTLS_PORT properties.put("mail.smtp.port", STARTTLS_PORT); // AUTH enabled properties.put("mail.smtp.auth", AUTH); // STARTTLS enabled properties.put("mail.smtp.starttls.enable", STARTTLS); // Authenticating Authenticator auth = new Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(USERNAME, PASSWORD); } }; // creating session session = Session.getInstance(properties, auth); // create mimemessage mimeMessage = new MimeMessage(session); //from address should exist in the domain mimeMessage.setFrom(new InternetAddress(FromAddress)); mimeMessage.addRecipient(RecipientType.TO, new InternetAddress(ToAddress)); mimeMessage.setSubject(EmailSubject); // setting text message body mimeMessage.setText(EmailBody); // setting HTML message body //mimeMessage.setContent(EmailBody,"text/html; charset=utf-8"); // sending mail Transport.send(mimeMessage); System.out.println("Mail Send Successfully"); } catch (Exception e) { e.printStackTrace(); } } }
Get the complete source code from Github
Thank you for the reading article. Please give comments, share and subscribe to get latest updates.
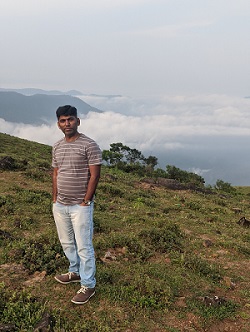
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.