How to decode Id Token in java
Table of Contents
Id Token generated by the authorization server which contains the user information and authorization server details.
Here I am sharing the java example program to decode the Id Token.
Pre-Requisites
- java 1.8
- Eclipse
Create java maven project in eclipse the add the following pom.xml and java file into your project and execute the program to decoded data of Id Token
POM.xml
Add the following dependency to POM.xml
<dependency> <groupId>com.auth0</groupId> <artifactId>java-jwt</artifactId> <version>3.2.0</version> </dependency>
Complete pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.narayanatutorial</groupId> <artifactId>DecodeIdToken</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>DecodeIdToken</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>com.auth0</groupId> <artifactId>java-jwt</artifactId> <version>3.2.0</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
DecodeIdToken.java
In the following sample program, I have given a sample ID Token to decode and test the program.
package com.narayanatutorial.DecodeIdToken; import com.auth0.jwt.JWT; import com.auth0.jwt.interfaces.DecodedJWT; public class DecodeIdToken { public static void main(String[] args) { //Give your Id Token to decrypt String id_token = "eyJ0eXAiOiJKV1QiLCJraWQiOiJ3VTNpZklJYUxPVUFSZVJCL0ZHNmVNMVAxUU09IiwiYWxnIjoiUlMyNTYifQ.eyJhdF9oYXNoIjoiOHNhdF9DdFJMM0FvUWFIZmM2RWdzZyIsInN1YiI6ImRlbW8iLCJhdWRpdFRyYWNraW5nSWQiOiJmMzczZjFlMC0xOTM0LTQxZGYtYmMwMy1kOWJmOGVmZDJkMmMtODE2Mjg3IiwiaXNzIjoiaHR0cDovL29wZW5hbS5uYXJheWFuYXR1dG9yaWFsLmNvbTo4OTkxL2FtL29hdXRoMiIsInRva2VuTmFtZSI6ImlkX3Rva2VuIiwiYXVkIjoibXlDbGllbnQiLCJjX2hhc2giOiJIamlnd0pxVzRNNjhSbE9OenZhQUtnIiwiYWNyIjoiMCIsIm9yZy5mb3JnZXJvY2sub3BlbmlkY29ubmVjdC5vcHMiOiJYajYyNnNFdmdzczQwdnQ3X3J3cEZmLXZzMmMiLCJzX2hhc2giOiJ1bmdXdjQ4QnotcEJRVURlWGE0aUl3IiwiYXpwIjoibXlDbGllbnQiLCJhdXRoX3RpbWUiOjE1ODYyMzM0MDUsInJlYWxtIjoiLyIsImV4cCI6MTU4NjIzNzA0NiwidG9rZW5UeXBlIjoiSldUVG9rZW4iLCJpYXQiOjE1ODYyMzM0NDZ9.cOksurVzCToTmyMjsLVIEjG2NB1nemtZCH5HtQ66SRB-a0EZahad7ZnD4P1P6_k1JDJDCJukEmYuWw1uxh0yOU_HV7tguNVUGD4XkbImuDUTGkO3-PGczNXREWw4SNbQ-JutKwBuYDMslpLUgKmzt2afGnjvGuwRMcZ5IQKx5Ul_Dw_bLGRIUy_spL6fSDb9zsSv2wLaf3Jz_IuaD8ORY3lqeTusRtqM6L3WoUqR7srH8kpz30xuopu-l5t1rhd7wgVvH9AJtxHsiMVLLOAML2Slm7bzi0t24s0Jiv74vdKqTXIqNIYoqUDhUa212U4hP0QgMTiHYV5_oNyEo_KzkQ"; //decrypt id_token DecodedJWT jwt = JWT.decode(id_token); String iss=jwt.getClaim("iss").asString(); String sub = jwt.getClaim("sub").asString(); String aud=jwt.getClaim("aud").asString(); Long ext=jwt.getClaim("ext").asLong(); Long iat=jwt.getClaim("iat").asLong(); String nonce=jwt.getClaim("nonce").asString(); String name=jwt.getClaim("name").asString(); String picture=jwt.getClaim("picture").asString(); String tokenName=jwt.getClaim("tokenName").asString(); String realm=jwt.getClaim("realm").asString(); String tokenType=jwt.getClaim("tokenType").asString(); Long exp=jwt.getClaim("exp").asLong(); Long auth_time=jwt.getClaim("auth_time").asLong(); String auditTrackingId=jwt.getClaim("auditTrackingId").asString(); System.out.println("iss: \t" + iss); System.out.println("sub: \t" + sub); System.out.println("aud: \t" + aud); System.out.println("ext: \t" + ext); System.out.println("iat: \t" + iat); System.out.println("nonce: \t" + nonce); System.out.println("name: \t" + name); System.out.println("picture: \t" + picture); System.out.println("tokenName: \t" + tokenName); System.out.println("realm: \t" + realm); System.out.println("tokenType: \t" + tokenType); System.out.println("exp: \t" + exp); System.out.println("auth_time: \t" + auth_time); System.out.println("auditTrackingId: \t" + auditTrackingId); } }
Output
iss: http://openam.narayanatutorial.com:8991/am/oauth2 sub: demo aud: myClient ext: null iat: 1586233446 nonce: null name: null picture: null tokenName: id_token realm: / tokenType: JWTToken exp: 1586237046 auth_time: 1586233405 auditTrackingId: f373f1e0-1934-41df-bc03-d9bf8efd2d2c-816287
Get the complete source from GitHub
Thanks for the reading article. Please give comments and subscribe to get the latest updates.
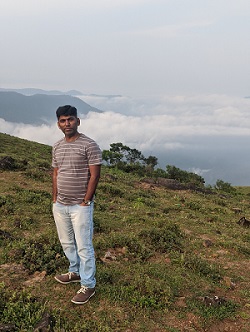
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.