Java SFTP Connection Shell Script Execute
Table of Contents [hide]
In this article, I am going to explain how to connect the SFTP server from Java API, how transfer files from the local system to remote SFTP server and how to execute remote shell scripts from java?
Pre-Requisites
- Java 1.8
- JSch.jar
- Eclipse IDE
- Maven
SftpShellChannel.java
To get SFTP connection, we need to get SFTP server configuration details as follows.
- SFTP Host/IP
- SFTP Port
- SFTP Username
- SFTP Password
jsch = new JSch(); session = jsch.getSession(USER, HOST, Integer.parseInt(PORT)); session.setConfig("StrictHostKeyChecking", "no"); session.setPassword(PASSWORD); session.connect(); channel = session.openChannel("shell");
Complete Code
package com.narayanatutorial.sftp; import com.jcraft.jsch.Channel; import com.jcraft.jsch.ChannelSftp; import com.jcraft.jsch.JSch; import com.jcraft.jsch.Session; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.FileWriter; import java.io.InputStream; import java.io.InputStreamReader; import java.io.OutputStream; import java.io.PrintStream; /** * * @author narayanatutorial */ public class SftpShellChannel { public static void main(String args[]) { Session session = null; ChannelSftp sftpchannel = null; Channel channel = null; JSch jsch = null; String USER = "TESTUSER"; String HOST = "127.0.0.1"; String PORT = "1523"; String PASSWORD = "TESTPASSWORD"; String SchellscriptPath = "/home/user/sample.sh"; String Param1 = "", Param2 = ""; try { jsch = new JSch(); session = jsch.getSession(USER, HOST, Integer.parseInt(PORT)); session.setConfig("StrictHostKeyChecking", "no"); session.setPassword(PASSWORD); session.connect(); channel = session.openChannel("shell"); if (channel.isConnected()) { System.out.println("SFTP Shell Channel Connected"); } else { System.out.println("SFTP Shell Channel Not Connected"); } channel.setOutputStream(null); //connection timout channel.connect(1000000); OutputStream inputstream_for_the_channel = channel.getOutputStream(); PrintStream commander = new PrintStream(inputstream_for_the_channel, true); // to execute shellscript commander.println("cd " + SchellscriptPath + " ; ./datapop_all.sh" + " " + Param1 + " " + Param2 + " ; exit"); commander.flush(); System.out.println("Exit Status:" + channel.getExitStatus()); //To read shellscript output and writing into log file InputStream outputstream_from_the_channel = channel.getInputStream(); BufferedReader br = new BufferedReader(new InputStreamReader(outputstream_from_the_channel)); String line = null; String LOGFILE = "D:/Debug.log"; while ((line = br.readLine()) != null) { System.out.println("Line:" + line); FileWriter fw = new FileWriter(LOGFILE, true); BufferedWriter bw = new BufferedWriter(fw); try { bw.write(line); bw.newLine(); } catch (Exception e) { System.out.println("Exception while creating logs..." + e.getMessage()); } finally { if (bw != null) { bw.close(); } if (fw != null) { fw.close(); } } } } catch (Exception e) { e.printStackTrace(); } finally { if (session.isConnected()) { session.disconnect(); } if (channel.isConnected()) { channel.disconnect(); } if (!sftpchannel.isConnected()) { sftpchannel.disconnect(); } } } }
pom.xml
pom.xml
<dependency> <groupId>com.jcraft</groupId> <artifactId>jsch</artifactId> <version>0.1.55</version> </dependency>
Complete pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.narayanatutorial.sftp</groupId> <artifactId>Java_SFTP</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>Java_SFTP</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <!-- https://mvnrepository.com/artifact/com.jcraft/jsch --> <dependency> <groupId>com.jcraft</groupId> <artifactId>jsch</artifactId> <version>0.1.55</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
Get complete source code from Github
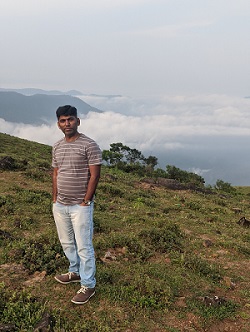
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.