Sometimes we need to open new popup window (browser) through javascript without disturbing main window. Popup windows are different to simply opening a new browser window. Here I am sharing different types of popup windows with different properties.
Popup window javascript syntax
Table of Contents
window.open(“url”, “window-Name”, “window-Features”);
url: Page url to open new windows,
window-Name : name of the window
window-Features: properties of the new popup window like width, height
Standard Popup Window
window.open (“https://narayanatutorial.com“,”mywindowName“);
Popup Window with toolbar
window.open (“https://narayanatutorial.com“,”mywindowName“, “toolbar=1“);
OR
window.open (“https://narayanatutorial.com“,”mywindowName“, “toolbar=yes“);
Popup Window with status
window.open (“https://narayanatutorial.com“,”mywindowName“, “status=1“);
OR
window.open (“https://narayanatutorial.com“,”mywindowName“, “status=yes“);
window.open (“https://narayanatutorial.com“,”mywindowName“, “menubar=1“);
Popup Window with width
window.open (“https://narayanatutorial.com“,”mywindowName“, “width=350“);
Popup Window with height
window.open (“https://narayanatutorial.com“,”mywindowName“, “height=350“);
Popup Window with resizable
window.open (“https://narayanatutorial.com“,”mywindowName“, “resizable=1“);
OR
window.open (“https://narayanatutorial.com“,”mywindowName“, “resizable=yes“);
Popup Window with scrollbars
window.open (“https://narayanatutorial.com“,”mywindowName“, “scrollbars=1“);
OR
window.open (“https://narayanatutorial.com“,”mywindowName“, “scrollbars=yes“);
Popup Window move to desired location
var my_new_window=window.open (“https://narayanatutorial.com“,”mywindowName“);
my_new_window.moveTo(0,0);
Popup Window with all windows features
var my_new_window= window.open(“https://narayanatutorial.com/“, “mywindowName“, “status=1,scrollbars=1,location=1,width=350,height=350“);
my_new_window.moveTo(0, 0);
Popup Window with all windows features parameterize
function popup(url)
{
var width=800;
var height=600;
var left = (screen.width - width)/2;
var top = (screen.height - height)/2;
var params = 'width='+width+', height='+height;
params += ', top='+top+', left='+left;
params += ', directories=no';
params += ', location=no';
params += ', menubar=no';
params += ', resizable=yes';
params += ', scrollbars=yes';
params += ', status=no';
params += ', toolbar=no';
newwin=window.open(url,'windowname5', params);
return false;
}
Above javascript function you can call from your html code as follows.
<html>
<head>
<title>JavaScript Popup Example 3</title>
</head>
<body onload="javascript: popup('https://narayanatutorial.com/')">
<h1>JavaScript Popup Example 3</h1>
</body>
</html>
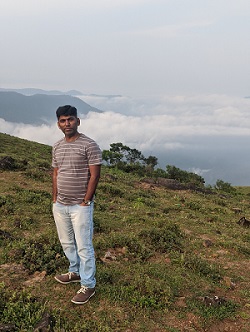
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.