This is example of run exe and batch files from java code and catch response from them in java.
Then create four java files in com.narayanatutorial.main package.
- RunExeMain.java
- RunWithParamMain.java
- RunBatchMain.java
- GetResponseMain.java
1) RunExeMain.java This file run exe file (Here cmd.exe) using java program. public class RunExeMain { //This is Example that display how to run exe using java public static void main(String args[]) { String filePath = "C:/Windows/cmd.exe"; try{ Process p = Runtime.getRuntime().exec(filePath); } catch(Exception e) { e.printStackTrace(); } } }
2) RunWithParamMain.java
This file run exe file and pass some parameter to it (Here we run notepad.exe and pass test.txt file to open) using java program.
public class RunWithParamMain { //This is Example that display how to run exe and pass parameter using java public static void main(String args[]) { String filePath = "C:/Windows/Notepad.exe D:/test.txt"; try { Process p = Runtime.getRuntime().exec(filePath); } catch (Exception e) { e.printStackTrace(); } } }
3) RunBatchMain.java
This file run batch file (Here test.bat) using java program.
public class RunBatchMain { //This is Example that display how to run batch using java public static void main(String args[]) { //Run batch file using java String filePath = "D:/test.bat"; try { Process p = Runtime.getRuntime().exec(filePath); } catch (Exception e) { e.printStackTrace(); } } }
4) GetResponseMain.java
This file run test.bat file and catch response from it using java program.
test.bat will contain the following data
@echo off
echo “this is test example”
public class GetResponseMain { public static void main(String args[]) { String filePath = "D:/test.bat"; try { Process p = Runtime.getRuntime().exec(filePath); p.waitFor(); InputStream in = p.getInputStream(); ByteArrayOutputStream baos = new ByteArrayOutputStream(); int c = -1; while((c = in.read()) != -1){ baos.write(c); } String response = new String(baos.toByteArray()); System.out.println("Response From Exe : "+response); } catch (Exception e) { e.printStackTrace(); } } } Output : Response From Exe : “this is test example”
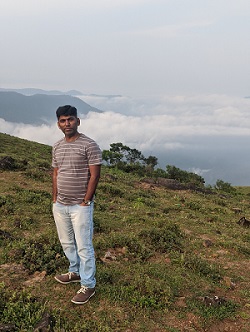
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.