Arrays Class
Table of Contents
This Class Present in java.util package. It defines several utility methods applicable for arrays.
Sorting the elements of Arrays:-Arrays class defines the following three methods to perform sorting.
- Public static void sort(Primitive[] p) to sort the elements of primitive array, according to natural sorting order.
- Public static void sort(Object[] o) to sort the elements of Object array, according to natural sorting order. If we are depending on natural sorting order, compulsory the objects should be homogeneous & comparable otherwise we will get ClassCastException.
- Public static void sort(Object[] o, Comparator()) to sort the elements of Object array, according to customized sorting order described by comparator object. we can sort Object Arrays either by natural sorting order or customized sorting order. But primitive arrays, we have to sort only by using natural sorting order.
MyComparator.java
package com.narayanatutorial.collections.arrays;
import java.util.Comparator;
class MyComparator implements Comparator
{
public int compare(Object o1, Object o2) {
// TODO Auto-generated method stub
String s1 = o1.toString();
String s2 = o2.toString();
return s2.compareTo(s1);
}
}
ArraysSortDemo.java
package com.narayanatutorial.collections.arrays;
import java.util.Arrays;
class ArraysSortDemo {
public static void main(String[] args) {
int[] a = { 10, 5, 20, 11, 6 };
System.out.println("Primitive Array before sorting");
for (int a1 : a) {
System.out.println(a1);
}
Arrays.sort(a);
System.out.println("Primitive Array after sorting");
for (int a1 : a) {
System.out.println(a1);
}
String[] s = { "A", "Z", "B" };
System.out.println("Object Array before sorting :");
for (String a1 : s) {
System.out.println(a1);
}
Arrays.sort(s);
System.out.println("Object Array after sorting");
for (String a2 : s) {
System.out.println(a2);
}
Arrays.sort(s, new MyComparator());
System.out.println("Object Array after sorting by comparator");
for (String a1 : s) {
System.out.println(a1);
}
}
}
Output
Primitive Array before sorting
10
5
20
11
6
Primitive Array after sorting
5
6
10
11
20
Object Array before sorting :
A
Z
B
Object Array after sorting
A
B
Z
Object Array after sorting by comparator
Z
B
A
Searching the Array
- Public static int binarySearch(PrimitiveArray[] P, Primitive key).
- Public static int LinearSearch(Object[]O, Object key).
- Public static int binarySearch(Object O, Object key Comparator c).
If the Array is sorted according to natural sorting Array, we have to use this method. If the Array is sorted according to comparator then we have to use this method.
Note:-
All the rules of Arrays Class binary search method are exactly same as collections class binarySearch method.
ArraysSearchDemo.java
package com.narayanatutorial.collections.arrays;
import java.util.Arrays;
public class ArraysSearchDemo {
public static void main(String[] args) {
int[] a = { 10, 5, 20, 11, 6 };
Arrays.sort(a); // sort by natural order
System.out.println(Arrays.binarySearch(a, 14)); // -5
String[] s = { "A", "Z", "B" };
System.out.println(Arrays.binarySearch(s, "C"));
Arrays.sort(s);
System.out.println(Arrays.binarySearch(s, "Z")); // 2
System.out.println(Arrays.binarySearch(s, "s")); // -4
Arrays.sort(s, new MyComparator2());
System.out.println(Arrays.binarySearch(a, new MyComparator2().compare("B", "A"))); // 2
System.out.println(Arrays.binarySearch(s, "C"));
}
}
ArraySearchemo2.java
package com.narayanatutorial.collections.arrays;
import java.util.Arrays;
public class ArraySearchemo2 {
public static void main(String arg[]) {
byte byteArr[] = { 10, 20, 15, 22, 35 };
char charArr[] = { 'g', 'p', 'q', 'c', 'i' };
int intArr[] = { 1, 2, 3, 4, 5, 6 };
double doubleArr[] = { 10.2, 15.1, 2.2, 3.5 };
float floatArr[] = { 10.2f, 15.1f, 2.2f, 3.5f };
short shortArr[] = { 10, 20, 15, 22, 35 };
Arrays.sort(byteArr);
Arrays.sort(charArr);
Arrays.sort(intArr);
Arrays.sort(doubleArr);
Arrays.sort(floatArr);
Arrays.sort(shortArr);
byte byteKey = 22;
char charKey = 'p';
int intKey = 3;
double doubleKey = 1.5;
float floatKey = 35;
short shortKey = 5;
System.out.println(byteKey + " found at index in byteArr = " + Arrays.binarySearch(byteArr, 2, 4, byteKey));
System.out.println(charKey + " found at index in charArr = " + Arrays.binarySearch(charArr, 1, 4, charKey));
System.out.println(intKey + " found at index in intArr = " + Arrays.binarySearch(intArr, 1, 4, intKey));
System.out.println(doubleKey + " found at index in doubleArr = " + Arrays.binarySearch(doubleArr, 1, 4, doubleKey));
System.out.println(floatKey + " found at index in floatArr = " + Arrays.binarySearch(floatArr, 1, 4, floatKey));
System.out.println(shortKey + " found at index in shortArr = " + Arrays.binarySearch(shortArr, 0, 4, shortKey));
}
}
output
22 found at index in byteArr = 3
p found at index in charArr = 3
3 found at index in intArr = 2
1.5 found at index in doubleArr = -2
35.0 found at index in floatArr = -5
5 found at index in shortArr = -1
Exceptions
- IllegalArgumentException : throws when starting index(fromIndex) is greater than the end index(toIndex) of specified range.(means: fromIndex > toIndex)
- ArrayIndexOutOfBoundsException : throws if one or both index are not valid means fromIndex<0 or toIndex > arr.length.
Important Points
If input list is not sorted, the results are undefined.
If there are duplicates, there is no guarantee which one will be found.
Converting Arrays to List
We can provide List view for the Array object, by using asList() method.
Public static List asList (object[] O):-
In this case, we are not creating an equivalent List object, just we are creating List reference for the existing Object Array.
By using List reference, we are perform any modification, it will be reflected in Array reference. Similarly by using Array reference if we are performing any modification, it will be reflected in List reference.
By using List reference we are not allow to Perform by operation which varies the size violation leads to Runtime Exception saying “UnsupportedOperationException”.
Reference Links
https://docs.oracle.com/javase/7/docs/api/java/util/Arrays.html#binarySearch(int[],%20int)
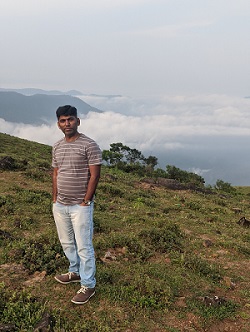
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.