Garbage Collection
Table of Contents
In C++, programmer is responsible for the creative of new objects& for destruction of useless objects Usually the programmer is taking very much car while creating object& he is ignoring that destruction of useless objects. Due to this negligence at cretin point of time, memory may not be available for the creation of new objects, as a result the whole application may fail due to memory problem.
But in java, the programmer is responsible only for the creation of object& sun people arranged one assistance for the destruction of useless object. This assistance always running in background& destroy useless object, because of the assistance, java program never fails due to memory problem. This assistance is nothing but Garbage collector, which is part of jvm.
Hence the main objective of Garbage collector is for destruction of useless object.
The way to make an object eligible for Garbage Collection:-
Even though programmer is not responsible for the destruction of object. It is always programming practice to make useless objects eligible for Garbage Collection. The following are different ways to make an eligible for Garbage Collection.
Nullifying the reference variable:-
If an object is no longer required, then assigned null to all its reference variable, then automatically that object is eligible for Garbage Collection.
student s1= new student();
student s2 = new student();
⇔ no object is eligible for Garbage Collection
s1 = null;
⇔ one object is eligible for Garbage Collection
s2 = null;
⇔ two objects are eligible for Garbage Collection
Reassigning the Reference variable:-
If an object is no longer required, assign all its reference variables to some other objects, the old object is by default eligible for Garbage Collection.
student s1= new student();⇔ no object is eligible for Garbage Collection
s1 = new student();⇔ one object is eligible for Garbage Collection
The objects created inside a method:-
The objects which are created inside a method are by default eligible for Garbage Collection once the method is completes.
Once the method is executes, the 2 objects are eligible for Garbage Collection.
Student.java
package com.narayanatutorial.collections.garbage;
public class Student {
public void getStudentDetails() {
// TODO Auto-generated method stub
}
public void setStudentDetails() {
// TODO Auto-generated method stub
}
}
GarbageTest.java
package com.narayanatutorial.collections.garbage;
public class GarbageTest {
public static void main(String[] args) {
m1();
}
public static void m1() {
Student s1 = new Student();
Student s2 = new Student();
}
}
GargbageTest1.java
package com.narayanatutorial.collections.garbage;
public class GarbageTest1 {
public static void main(String[] args) {
// TODO Auto-generated method stub
Student s3 = m1(); // one object is eligible for GC i.e s2
}
public static Student m1() {
Student s1 = new Student();
Student s2 = new Student();
return s1;
}
}
GarbageTest2.java
package com.narayanatutorial.collections.garbage;
public class GarbageTest12 {
public static void main(String[] args) {
// TODO Auto-generated method stub
m1(); // two object is eligible for GC i.e s1 and s2
}
public static Student m1() {
Student s1 = new Student();
Student s2 = new Student();
return s1;
}
}
GarbageTest3.java — Island of Isolation
package com.narayanatutorial.collections.garbage;
public class GarbageTest13 {
public static void main(String[] args) {
Student s;
// TODO Auto-generated method stub
Student s1=new Student();
Student s2=new Student();
Student s3=new Student();
s1=s2;
s2=s3;
s3=s1;
//No object eligible for Garbage Collection
s1=null;
s2=null;
s3=null;
//3 Objects eligible for Garbage Collection
}
}
The ways for requesting JVM to run Garbage Collector:-
https://www.oracle.com/webfolder/technetwork/tutorials/obe/java/gc01/index.html
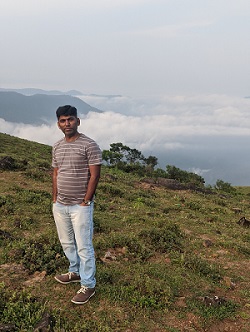
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.