Queue
Table of Contents
- This interface has introduced in 1.5 version.
- If we want to represent, group of Objects Prior to processing, then we should go for Queue.
- In general Queue follow FIFO, but we can customize based on our requirement.
*image*
- From 1.5 version on wards linkedList also implement Queue interface.
- LinkedList implementation of Queue always follows FirstInFirstOut.
Methods of Queue interface:-
- Boolean offer (object o )
- For adding an object to the Queue.
- Object Peek()
- Return head element of the Queue . If the Queue is empty this method returns null.
- Object elements().
- Returns head element of the Queue. If Queue is empty, this method raises RuntimeException saying NoSuchElementException.
- Object remove()
- Remove & returns head element of the Queue. If the Queue is empty, this method returns null.
- Object remove()
- Remove & returns head element of the Queue. If Queue is empty, this method raises NoSuchElementException.
Priority Queue
- It represents a data structure to hold a group of individual Objects prior to processing based on same priority.
- It can be default natural sorting order or customized sorting order specified by comparator object.
- If we are depending on default natural sorting order then objects should be homogeneous & comparable, otherwise we will get ClassCastException.
- If we are defining our own sorting order, by comparator, then the objects need not be Homogeneous comparable.
- Insertion order is not preserve.
- Duplicate objects are not allow.
- null insertion is not possible, even as the first element also.
Constructor
PriorityQueue q = new PrioryQueue()
- Creates an empty priorityQueue with default initial capacity 11 & sorting order in natural sorting order.
- PriorityQueue q = new PriorityQueue(int initialcapacity);
- PriorityQueue q = new PriorityQueue (int initialcapacity, Comparator c);
- PriorityQueue q = new PriorityQueue (SortedSet s). // some platforms may not provide the support for PriorityQueues properly.
PriorityQueueDemo1.java
package com.narayanatutorial.collections.queue;
import java.util.NoSuchElementException;
import java.util.PriorityQueue;
public class PriorityQueueDemo1 {
public static void main(String[] args) {
// TODO Auto-generated method stub
PriorityQueue<Integer> q = new PriorityQueue<Integer>();
System.out.println(q.peek());// null
try {
System.out.println(q.element()); // NoSuchElementException
}catch(NoSuchElementException e) {
System.out.println("No Such Element Exception.."+e);
}
for (int i = 0; i <= 10; i++) {
q.offer(i);
}
System.out.println("PriorityQueue:"+q);// 10,1,2,3,....10
System.out.println("poll:"+q.poll()); // 0
System.out.println("PriorityQueue:"+q); // [1,2,3,4.....10]
}
}
Output
null
No Such Element Exception..java.util.NoSuchElementException
PriorityQueue:[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
poll:0
PriorityQueue:[1, 3, 2, 7, 4, 5, 6, 10, 8, 9]
Mycomparator.java
package com.narayanatutorial.collections.queue;
import java.util.Comparator;
public class Mycomparator implements Comparator<Object> {
@Override
public int compare(Object obj1, Object obj2) {
// TODO Auto-generated method stub
String s1 = (String) obj1;
String s2 = (String) obj2;
return s2.compareTo(s1);
}
}
PriorityQueueDemo2.java
package com.narayanatutorial.collections.queue;
import java.util.PriorityQueue;
public class PriorityQueueDemo2 {
public static void main(String[] args) {
// TODO Auto-generated method stub
PriorityQueue<String> q =new PriorityQueue<String>(new Mycomparator());
q.offer("A");
q.offer("B");
q.offer("Z");
q.offer("L");
System.out.println("customize sorting order:"+q); // [ZLBA]
PriorityQueue<String> q1 =new PriorityQueue<String>(new Mycomparator().reversed());
q1.offer("A");
q1.offer("B");
q1.offer("Z");
q1.offer("L");
System.out.println("customize sorting order with reversed method of comparator:"+q1); // [ZLBA]
}
}
Output
customize sorting order:[Z, L, B, A]
customize sorting order with reversed method of comparator:[A, B, Z, L]
Reference Links
https://docs.oracle.com/javase/6/docs/api/
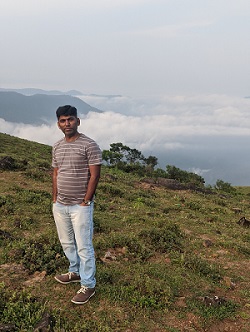
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.