CSV File Read Data Into Bean Object
Table of Contents
I am going to explaining about CSV File Read Data Into Bean Object. In which explaining how to read csv file data and putting into bean object which is nothing but view object(VO).
You can see what is CSV file?.
Before going to write java program we need to download opencsv jar file.
Find the below sample data and save it in the format of csv file extension.
How to create csv file
- Open note pad
- Paste the below data into note pad
- Save it as like “OpenCsvRead.csv” in the double quote as file name.
FIRSTNAME,LASTNAME,AGE Narayana,Ragi,30 Kumar,Chitra,50 Swamy,Bathala,40
OpenCsvReadToBean.java
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package com.narayanatutorial.opencsv; import au.com.bytecode.opencsv.CSVReader; import au.com.bytecode.opencsv.bean.ColumnPositionMappingStrategy; import au.com.bytecode.opencsv.bean.CsvToBean; import java.io.FileReader; import java.util.List; public class OpenCsvReadToBean { public static void main(String args[]) { String csvFilename = "D:/narayanatutorial/SampleFiles/OpenCSVRead.csv"; try { ColumnPositionMappingStrategy cpm = new ColumnPositionMappingStrategy(); cpm.setType(Profile.class); String[] csvcolumns = new String[]{"firstName", "lastName", "age"}; cpm.setColumnMapping(csvcolumns); CSVReader csvReader = new CSVReader(new FileReader(csvFilename)); CsvToBean csvtobean = new CsvToBean(); List csvDataList = csvtobean.parse(cpm, csvReader); for (Object dataobject : csvDataList) { Profile profile = (Profile) dataobject; System.out.println("Firstname:"+profile.getFirstName()+ " LastName:"+profile.getLastName()+" Age:"+profile.getAge()); } } catch (Exception e) { System.out.println("exception :" + e.getMessage()); } } }
Output
Firstname:FIRSTNAME LastName:LASTNAME Age:AGE Firstname:Narayana LastName:Ragi Age:30 Firstname:Kumar LastName:Chitra Age:50 Firstname:Swamy LastName:Bathala Age:40
Explanation
Set Header and Assign Bean Class
ColumnPositionMappingStrategy cpm = new ColumnPositionMappingStrategy(); cpm.setType(Profile.class); String[] csvcolumns = new String[]{"firstName", "lastName", "age"}; cpm.setColumnMapping(csvcolumns);
ColumnPositionMappingStrategy class will be used to assign the bean / VO (Profile) class and set the column mapping as string array. The column mapping string array and profile class parameters should be same. And profile class contains setter and getter methods of each parameters.
Reading CSV file
CSVReader csvReader = new CSVReader(new FileReader(csvFilename));
Split CSV file As per the Assigned Header and return list
CsvToBean csvtobean = new CsvToBean(); List csvDataList = csvtobean.parse(cpm, csvReader);
Iterating list and assigning values to Profile class
for (Object dataobject : csvDataList) { Profile profile = (Profile) dataobject; System.out.println("Firstname:"+profile.getFirstName()+" LastName:" +profile.getLastName()+" Age:"+profile.getAge()); }
you can find the the sample program in the git. If you have git account you can download from git and also other sample programs also available and find the below git link to download. We used Netbeans IDE to develop sample programs. So you can directly import into Netbeans if you are having else create sample project in your IDE and replace src folder.
Git link
I hope you understand CSV File Read Data Into Bean Object by using opencsv jar file.
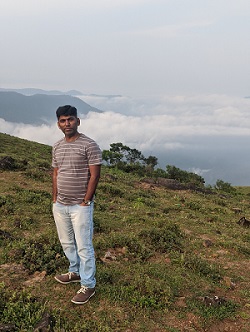
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.