CSV File Read All Lines
Table of Contents
In the post I am going to explaining CSV file read all lines by using opencsv jar file. You can see what is CSV file?.
Before going to write java program we need to download opencsv jar file.
Find the below sample data and save it in the format of csv file extension.
How to create csv file
- Open note pad
- Paste the below data into note pad
- Save it as like “OpenCsvRead.csv” in the double quote as file name.
FIRSTNAME,LASTNAME,AGE Narayana,Ragi,30 Kumar,Chitra,50 Swamy,Bathala,40
OpenCsvReadAll.java
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.narayanatutorial.opencsv;
import au.com.bytecode.opencsv.CSVReader;
import java.io.FileReader;
import java.util.List;
public class OpenCsvReadAll {
public static void main(String args[]) {
String csvFilename = "D:/narayanatutorial/SampleFiles/OpenCSVRead.csv";
String[] csvRow = null;
try {
CSVReader csvReader = new CSVReader(new FileReader(csvFilename));
/* If you want to parse a file with other delimiter like semicolon (;)
or hash (#), you can do so by calling a different constructor of CSVReader class:
*/
//CSVReader csvReader = new CSVReader(new FileReader(csvFilename), ';');
//CSVReader csvReader = new CSVReader(new FileReader(csvFilename), '#');
//CSVReader csvReader = new CSVReader(new FileReader(csvFilename), ',');
/* CSV file’s value is quoted with single quote (‘) instead of default double quote (“) */
// CSVReader csvReader = new CSVReader(new FileReader(csvFilename), ',', '\'');
/* reader will skip 5 lines from top of CSV and starts processing at line 6*/
//CSVReader csvReader = new CSVReader(new FileReader(csvFilename), ',', '\'', 1);
List<String[]> csvAllRows = csvReader.readAll();
for (Object object : csvAllRows) {
csvRow = (String[]) object;
System.out.println(csvRow[0] + " # " + csvRow[1] + " # " + csvRow[2]);
}
} catch (Exception e) {
System.out.println("exception :" + e.getMessage());
}
}
}
Output
FIRSTNAME # LASTNAME # AGE Narayana # Ragi # 30 Kumar # Chitra # 50 Swamy # Bathala # 40
Explanation
List<String[]> csvAllRows = csvReader.readAll();
for (Object object : csvAllRows) {
csvRow = (String[]) object;
System.out.println(csvRow[0] + " # " + csvRow[1] + " # " + csvRow[2]);
}
- readAll() method highlighted in blue color will read all lines of csv file and putting into list as row and each row split by delimiter into string array.
- While list retrieve, it will return string array for each iteration
- Each string array contains one csv row and string array we can retrieve by using index position
Constructor
CSV Reader class having different types of constructors as follows.
new CSVReader(new FileReader(csvFilename));
The above constructor will read csv file with FileReader as parameter,
Reading CSV file and Splitting
//CSVReader csvReader = new CSVReader(new FileReader(csvFilename), ';'); //CSVReader csvReader = new CSVReader(new FileReader(csvFilename), '#'); //CSVReader csvReader = new CSVReader(new FileReader(csvFilename), ',');
The above constructor will read csv file and splitting as we specified delimiter in the second parameter.
Remove Single / Double Quotes
/* CSV file’s value is quoted with single quote (‘) instead of default double quote (“) */ // CSVReader csvReader = new CSVReader(new FileReader(csvFilename), ',', '\'');
The above constructor will read csv file and splitting as we specified delimiter in the second parameter and removing the single quotes for each value. Find the below sample input format for the above constructor.
'FIRSTNAME','LASTNAME','AGE' 'Narayana','Ragi','30' 'Kumar','Chitra','50' 'Swamy','Bathala','40'
Remove CSV Header
/* reader will skip 1 line from top of CSV and starts processing at line 2*/ //CSVReader csvReader = new CSVReader(new FileReader(csvFilename), ',', '\'', 1);
The above constructor will read file and skip number of lines we specified in the 4th parameter and start the reading the file from +1 row. i.e n number of line skipped and will read from n+1 row.
Sample input data
'FIRSTNAME','LASTNAME','AGE' 'Narayana','Ragi','30' 'Kumar','Chitra','50' 'Swamy','Bathala','40'
Output
'Narayana','Ragi','30' 'Kumar','Chitra','50' 'Swamy','Bathala','40'
you can find the above sample program in the github. If you have git account you can download from github and other sample programs also available and find the below github link to download. We used Netbeans IDE to develop sample programs. So you can directly import into Netbeans if you are having Netbeans else create sample project in your IDE and replace src folder.
Git link
I hope you understand CSV File Read All Lines by using opencsv jar file.
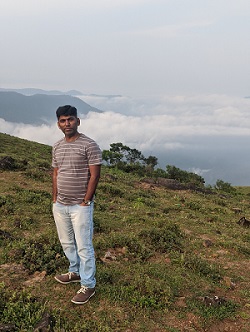
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.