Spring Boot Scheduler
Table of Contents
In this article, we will discuss Schedule configuration in Spring Boot. By using @Scheduled annotations, you can easily enable the scheduler with different types of schedule execution. Schedule enabled method should not accept any method parameter and method return should be void.
Enable Spring Boot Scheduler
By using @EnableScheduling annotation, you can enable spring boot schedule
@EnableScheduling
@SpringBootApplication
public class SpringBootSchedulerApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootSchedulerApplication.class, args);
}
}
Enable Spring Boot Fixed Delay Scheduler
By using @Scheduled(fixedDelay = 10), you can enable a fixed delay scheduler. It means that its delay time in n milliseconds after completion of the task before starting the next task.
Here the mentioned the number in milliseconds.
@Scheduled(fixedDelay = 10)
public void fixedDelaySchedulerMethod() {
System.out.println("fixedDelaySchedulerMethod ..execution.");
}
Enable Spring Boot Fixed Rate Scheduler
By using @Scheduled(fixedRate = 10), you can enable fixedRate schedule. It means that every 10millisec will be executed independently but the next schedule task will be executed after the completion of the first task. Its sequential process, not a parallel process.
@Scheduled(fixedRate = 10)
public void fixedRateSchedulerMethod() {
System.out.println("fixedRateSchedulerMethod ..execution.");
}
It’s similar to FixedDelay but to make it parallel scheduler task execution then you have to enable async scheduler.
The below method will be executed every 10 milliseconds in parallel, the next scheduler task execution will not wait to first scheduler task completion.
@Async @Scheduled(fixedRate = 10) public void fixedRateSchedulerAsyncMethod() { System.out.println("fixedRateSchedulerAsyncMethod ..execution."); }
Enable Spring Boot Initial Delay Scheduler
By using initialDelay, you can enable a fixed delay schedule. It means that the scheduled task will be executed at 10 milliseconds after the application started.
Suppose we have a requirement like execute the schedule after 10 milliseconds since application up and running.
@Scheduled(fixedRate = 10, initialDelay = 10)
public void initialDelaySchedulerMethod() {
System.out.println("initialDelaySchedulerMethod ..execution.");
}
Enable Spring Boot cron Job Scheduler
By using @Scheduled(cron = “0/30 * * * * ? *”), you can enable cron job scheduler. Cron job expression is easily configurable
@Scheduled(cron = "0/30 * * * * ? *")
public void cronSchedulerMethod() {
System.out.println("cronSchedulerMethod ..execution.");
}
Enable Spring Boot Parameterized Scheduler
Schedule interval time can be parameterized in the application.properties file instead of hard code in the java file.
Fixed Dealy
Add the fixedDelay.in.milliseconds=10 in application.properties file
@Scheduled(fixedDelayString = "${fixedDelay.in.milliseconds}")
public void fixedDelaySchedulerStringMethod() {
System.out.println("fixedDelaySchedulerStringMethod ..execution.");
}
Fixed Rate
Add the fixedRate.in.milliseconds=10 in application.properties file
@Scheduled(fixedRateString = "${fixedRate.in.milliseconds}")
public void fixedRateSchedulerStringMethod() {
System.out.println("fixedRateSchedulerStringMethod ..execution.");
}
Get complete source code from GitHub
Thank you for reading the article. Please write the comments and subscribe to get the latest updates.
References
http://www.quartz-scheduler.org/documentation/quartz-2.3.0/tutorials/crontrigger.html
https://docs.oracle.com/cd/E12058_01/doc/doc.1014/e12030/cron_expressions.htm
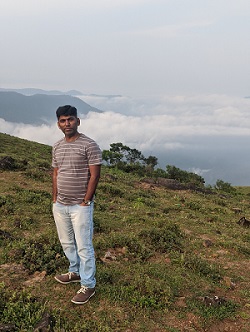
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.