Static Import
Table of Contents
This concept is introduces in 1.5, According to static import, improves readability of the code. But According to world wide experts, static import reduces readability and increases the confusion of the code. Hence if there is no specific requirement, it is not recommended to use static import.
When we are using static import, it is not required to use class name, while accessing static members.
With out static import:-
import java.lang.* ; Class test Public static void main (String[] args) { System.out.println (Math.sqrt (4)); System.out.println (Math.randam ()); System.out.println(Math.max (10, 20)); } }
With static import:-
import static java.lang.Math.sqrt; import static java.lang.Math.*; Class test Public static void main( String[] args) { System.out.println (sqrt (4)); System.out.println (sqrt ()); System.out.println (max(10, 20)); } }
import static java.lang.Byte.*; import static java.lang.short.*; Class test { Public static void main (String[] args) { System.out.println (MAX_VALUE); } }
While resolving static members, compile will always gives precedence in the following order.
- Current class static members.
- Explicit static import.
- Implicit static import.
import static java.lang.Integer.MAX_VALUE; import static java.lang.Byte.*; Class test { static int MAX_VALUE = 888; Public static void main (String[] args) { System.out.println(MAX_VALUE); } }
- If we are commenting line 1, explicit static import will be consider and we will Integer class MAX_VALUE is 2147483647.
- If we are commenting line 1 and 2, then byte class MAX_VALUE will be consider and 127 as the output.
- It is never recommended to use import concept until method level or variable level. But we have to use only at class level i.e general import use only at class level i.e. general import recommended to use, but not static import.
Que:- Which of the following import statements are not valid ?
- import static java.util.*;
- import static java.util.ArrayList ;
- import java.util.ArrayList ;
- import static java.util.lang.Math.sqrt ;
- import java.util.ArrayList.* ;
- import java.util.lang.Math.sqrt ;
- import static java.util.lang.Math.* ;
- import java.util.lang.Math.* ;
Ans:- 1, 2, 4 .
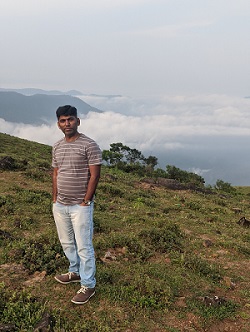
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.