How to Send Email Using Java Gmail SMTP With SSL
Table of Contents
In this article, I am going to explaining How to Send Email Using Java Gmail SMTP With SSL. SSL is a secure layer. To send email insecurely, have to use Gmail SSL port to establish a Gmail SMTP connection.
In the following, sending an email text body by using java Gmail SMTP With SSL.
Gmail SMTP SSL Port: 465
Gmail SSL Code
properties.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory");
Pre-requisites
- 1. Java 1.8
- 2. Eclipse IDE
In eclipse, Create maven project and add the Java mail API dependency jar in pom.xml as follows
POM.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.narayanatutorial</groupId> <artifactId>JavaMail</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>JavaMail</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <!-- https://mvnrepository.com/artifact/javax.mail/mail --> <dependency> <groupId>javax.mail</groupId> <artifactId>mail</artifactId> <version>1.4.7</version> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
GmailTextMsgSendWithSSL.java
package com.narayanatutorial.gmail; import java.util.Properties; import javax.mail.Authenticator; import javax.mail.Message.RecipientType; import javax.mail.MessagingException; import javax.mail.PasswordAuthentication; import javax.mail.Session; import javax.mail.Transport; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeMessage; public class GmailTextMsgSendWithSSL { Properties properties; Session session; MimeMessage mimeMessage; String USERNAME = "[email protected]"; String PASSWORD = "XXXXXX"; String HOSTNAME = "smtp.gmail.com"; String SSL_PORT = "465"; boolean AUTH = true; String SERVERTYPE = "smtp"; public static void main(String args[]) throws MessagingException { String EmailSubject = "Subject:Text Subject"; String EmailBody = "Text Message Body: Hello World"; String ToAddress = "[email protected]"; GmailTextMsgSendWithSSL gmailTextMsgSend = new GmailTextMsgSendWithSSL(); gmailTextMsgSend.sendGmail(EmailSubject, EmailBody, ToAddress); } public void sendGmail(String EmailSubject, String EmailBody, String ToAddress) { try { properties = new Properties(); properties.put("mail.smtp.host", HOSTNAME); // SSL_PORT Enabled properties.put("mail.smtp.port", SSL_PORT); // AUTH Enabled properties.put("mail.smtp.auth", AUTH); // SSL Enabled properties.put("mail.smtp.socketFactory.port", SSL_PORT); properties.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory"); // Authenticating Authenticator auth = new Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(USERNAME, PASSWORD); } }; // creating session session = Session.getDefaultInstance(properties, auth); // create mimemessage mimeMessage = new MimeMessage(session); mimeMessage.addRecipient(RecipientType.TO, new InternetAddress(ToAddress)); mimeMessage.setSubject(EmailSubject); // setting text message body mimeMessage.setText(EmailBody); // sending mail Transport.send(mimeMessage); System.out.println("Mail Send Successfully"); } catch (Exception e) { e.printStackTrace(); } } }
Some other important links
Gmail SMTP SSL – Sending Email Using JavaMail API
How to send HTML Email Body Using Java Gmail SMTP
Thanks for reading. You can subscribe to get more updates.
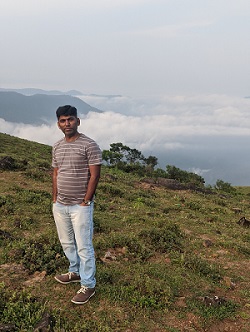
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.