We are going to explaining What is throw,throws,finally in java?
throws
- throws clause in used to declare an exception and postpone the handling of a checked exception
- throws clause is used to declare checked and un-checked exceptions
- throws clause is followed by exception class names.
- throws clause is used in method
Syntax
public void getData() throws FileNotFoundException{ //To do something }
- We can declare multiple exceptions by using throws clause
Syntax
public void getData() throws IOException, FileNotFoundException, SQLException { //To do something }
throw
- throw keyword is used to throw an exception explicitly.
- throw is followed by an instance variable
- throw is used inside method body to invoke an exception
Syntax
public void getData(){ try { throw new Exception("Exception occured."); } catch (Exception exp) { System.out.println("Error: "+exp.getMessage()); } }
- We cannot throw more than one exception by using throw clause
Syntax
throw new ArithmeticException("An integer should not be divided by zero!!") throw new IOException("Connection failed!!")
Finally
- The finally block follows a try block or a catch block.
Syntax
Combination 1 try{ //To do something }finally{ //To do something } Combination 2 try{ //To do something }catch(Exception e){ //To do something }finally{ //To do something } Combination 3 try{ //To do something }catch(ExceptionType1 e1){ //To do something }catch(ExceptionType2 e2){ //To do something }catch(ExceptionType3 e3){ //To do something }finally{ //To do somehting }
- Using a finally block allows you to run any cleanup-type statements, closing connections and resources, releasing memory even if we got exception in the method.
- A finally block of code always executes, irrespective of occurrence of an Exception.
- A finally of code will not executes in some cases like System.exit(0), System.exit(1). These statements will terminate the program forcefully and control will not reach finally block.
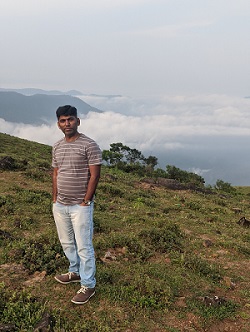
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.