Java Reflection API
Table of Contents
Java Reflection API is a process of modifying or retrieve metadata of a class at run time.
The java.lang.Class class provides methods that can be used to get metadata and change the run time behavior of a class.
The java.lang and java.lang.reflect packages are providing classes for java reflection.
The Java Reflection API is using mainly as follows.
- IDE Development (Example: Eclipse, Myeclipse, Netbeans etc…)
- Testing Tools
- Debugger Tools
How to create Object of java.lang.Class?
- Java.lang.Class provides methods to get metadata at a class runtime.
- Java.lang.Class provides methods to change the runtime behavior of the class.
There are 3 ways to create the Instance of the Class class
- forName() method of Class class
- getClass() method of Object class
- the .class syntax
forName() method of Class class
- It is used to load the Class dynamically
- It retruns instance of the Class
- It should be used, if we know the fully qaulified class name
- It can not be used for primitive types
Example :
package com.samples; public class NTutorial{ } package com.samples; public class Tutorial{ public static void main(String args[]) throws ClassNotFoundException{ Class c=Class.forName("com.samples.NTutorial"); System.out.println("Class name:"+c.getName()); } } Output Class name:com.samples.NTutorial
getClass() method of Object class
- It returns the instance of Class class.
- It should be used if you know the type.
- It can be used with primitives.
Example :
package com.samples; public class NTutorial{ } package com.samples; public class Tutorial{ public void display(Object obj){ Class cls=obj.getClass(); System.out.println("Class Name:"+cls.getName()); } public static void main(String args[]) { NTutorial nt=new NTutorial(); Tutorial t=new Tutorial(); t.display(nt); } } Output Class Name: NTutorial
the .class syntax
- It can be used like appending “.class” to type as follows in the example
- It can be used for primitive data type also.
Example :
public class Tutorial{ public static void main(String args[]) { Class c1=boolean.class; Class c2=int.class; Class c3=char.class; Class c4=double.class; Class c5=Tutorial.class; System.out.println(c1.getName()); System.out.println(c2.getName()); System.out.println(c3.getName()); System.out.println(c4.getName()); System.out.println(c5.getName()); } } Output boolean int char double Tutorial
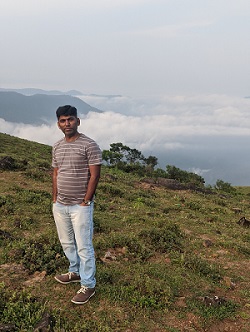
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.