JKS certificate using for accessing web application through secure https protocol instead http. Assume JKS file is created but you didn’t remember some of the key details to setup in the server level like key store type, key store provider, creation date, alias name, validity, owner etc.. This article helps you to get this information.
To know basic information of JKS file
Table of Contents
First of all open command prompt and then go to JKs file location and then execute the following command. After press enter, it will ask password, so enter the password and then press enter.
Syntax
C:\Users\narayanatutuorial> keytool -list -keystore <JKS file name>
Example
C:\Users\narayanatutuorial> keytool -list -keystore keystore-narayanatutorial.jks Enter keystore password: Keystore type: JKS Keystore provider: SUN Your keystore contains 1 entry selfsigned, 30 Nov, 2017, PrivateKeyEntry, Certificate fingerprint (SHA1): C8:BE:B7:89:BF:36:CB:62:B8:25:9F:34:C2:53:F8:11: 90:2A:A2:B3
To know alias name correct or not of JKS file
First of all open command prompt and then go to JKs file location and then execute the following command. After press enter, it will ask password, so enter the password and then press enter.
Syntax
C:\Users\narayanatutuorial> keytool -list -keystore <JKS file name> -alias <alias name>
Example
Wrong Alias name if entered
C:\Users\narayanatutuorial> keytool -list -keystore keystore-narayanatutorial.jks -alias narayana Enter keystore password: keytool error: java.lang.Exception: Alias <narayana> does not exist
Correct Alias name if entered
C:\Users\narayanatutuorial> keytool -list -keystore keystore-narayanatutorial.jks -alias narayanatutorial Enter keystore password: narayanatutorial, 30 Nov, 2017, PrivateKeyEntry, Certificate fingerprint (SHA1): C8:BE:B7:89:BF:36:CB:62:B8:25:9F:34:C2:53:F8:11: 90:2A:A2:B3
To know complete information of JKS file
First of all open command prompt and then go to JKs file location and then execute the following command. After press enter, it will ask password, so enter the password and then press enter.
Syantax
C:\Users\narayanatutuorial> keytool -v -list -keystore <JKS file name>
Example
C:\Users\narayanatutuorial> keytool -v -list -keystore keystore-narayanatutorial.jks Enter keystore password: Keystore type: JKS Keystore provider: SUN Your keystore contains 1 entry Alias name: narayanatutorial Creation date: 30 Nov, 2017 Entry type: PrivateKeyEntry Certificate chain length: 1 Certificate[1]: Owner: CN=narayanatutorial, OU=narayanatutorial, O=narayanatutorial, L=bangalore, ST=karnataka, C=in Issuer: CN=narayanatutorial, OU=narayanatutorial, O=narayanatutorial, L=bangalore, ST=karnataka, C=in Serial number: 46c67a5 Valid from: Thu Nov 30 18:07:07 IST 2017 until: Sun Nov 25 18:07:07 IST 2018 Certificate fingerprints: MD5: 02:B5:87:66:B3:38:75:BC:B4:5D:26:6F:96:B1:62:15 SHA1: C8:BE:B7:89:BF:36:CB:62:B8:25:9F:34:C2:53:F8:11:90:2A:A2:B3 SHA256: C0:DC:5C:0F:DC:BD:37:A5:FB:8D:15:89:C8:E6:FA:10:A3:29:36:B0:AD: 91:FB:8B:52:34:FE:D9:60:84:92:82 Signature algorithm name: SHA256withRSA Version: 3 Extensions: #1: ObjectId: 2.5.29.14 Criticality=false SubjectKeyIdentifier [ KeyIdentifier [ 0000: B7 F2 A2 25 9B A7 0D B0 AD 0E FC EA 77 CF 15 8F ...%........w... 0010: 84 73 37 CA .s7. ] ] ******************************************* *******************************************
To know complete information of JKS file by using java program
package JKS; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.security.KeyStore; import java.security.KeyStoreException; import java.security.NoSuchAlgorithmException; import java.security.cert.Certificate; import java.util.Enumeration; public class JKSInfo { public static void main(String args[]) throws FileNotFoundException, KeyStoreException, IOException, NoSuchAlgorithmException { FileInputStream is = null; try { File file = new File(""); is = new FileInputStream("D:/narayanatutorial/keystore.jks"); KeyStore keystore = KeyStore.getInstance(KeyStore.getDefaultType()); String password = "password"; keystore.load(is, password.toCharArray()); Enumeration enumeration = keystore.aliases(); while (enumeration.hasMoreElements()) { String alias = (String) enumeration.nextElement(); System.out.println("alias name: " + alias); Certificate certificate = keystore.getCertificate(alias); System.out.println("Informatoin: "+certificate.toString()); } } catch (java.security.cert.CertificateException e) { e.printStackTrace(); } catch (NoSuchAlgorithmException e) { e.printStackTrace(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (KeyStoreException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally { if (null != is) { try { is.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
Output
alias name: narayanatutorial Informatoin: [ [ Version: V3 Subject: CN=narayanatutorial, OU=narayanatutorial, O=narayanatutorial, L=bangalore, ST=karnataka, C=in Signature Algorithm: SHA256withRSA, OID = 1.2.840.113549.1.1.11 Key: Sun RSA public key, 2048 bits modulus: 21606185906718923752804871503489161479488473142379754057540478038758185768954186129883696338159491500025146543947988439689990247356932355932019221134014212738731267172073913172974911172313919063053365992273221799299400937323088194743356879676842999243013402405395508887161417043395729762256908680498801665903269609812997275103259467950444678347257078380603776674199965496764303535104168335784296717774788887314803960495123999003193982695425527540134398208594824079235535802619456363450464509418988379824005568486090383886649356975851819785995113839637185861107896210761525435700549170944058589771281515153602805167441 public exponent: 65537 Validity: [From: Thu Nov 30 18:07:07 IST 2017, To: Sun Nov 25 18:07:07 IST 2018] Issuer: CN=narayanatutorial, OU=narayanatutorial, O=narayanatutorial, L=bangalore, ST=karnataka, C=in SerialNumber: [ 046c67a5] Certificate Extensions: 1 [1]: ObjectId: 2.5.29.14 Criticality=false SubjectKeyIdentifier [ KeyIdentifier [ 0000: B7 F2 A2 25 9B A7 0D B0 AD 0E FC EA 77 CF 15 8F ...%........w... 0010: 84 73 37 CA .s7. ] ] ] Algorithm: [SHA256withRSA] Signature: 0000: 01 A7 98 D9 15 FE 52 49 75 79 CD AC F9 1C FE 8F ......RIuy...... 0010: D7 10 7D 67 75 8E 8A 7B 61 6B 28 E6 8F 04 B8 AE ...gu...ak(..... 0020: 15 DF 19 D9 50 D3 FD E1 EE FB F7 6D 70 6C 69 CA ....P......mpli. 0030: B9 5F 90 77 5A B9 DA 23 AE 51 FA A1 2A DE 01 BB ._.wZ..#.Q..*... 0040: 24 1F 15 38 63 2F 8A 13 5C 58 3A 7F F2 5A EC 94 $..8c/..\X:..Z.. 0050: 7D E9 2A 67 34 20 23 8D 5D 56 0E 43 9C FB 92 3C ..*g4 #.]V.C...< 0060: 5A 3C CB 34 E0 0A 6A 80 77 B5 F4 E4 00 26 20 71 Z<.4..j.w....& q 0070: 1E 0C D9 9E 54 37 F0 94 DD 44 A6 49 F5 34 53 56 ....T7...D.I.4SV 0080: 4A DB 03 3F B6 EF 8D 33 F1 A6 BC A4 CE CD E5 CB J..?...3........ 0090: BD 35 DD 67 6B 8E 72 76 2C 38 E8 BE 4A 03 5E 48 .5.gk.rv,8..J.^H 00A0: CC 5D 10 63 83 EC AD F2 EE 31 D6 B2 7D A9 44 84 .].c.....1....D. 00B0: 66 63 E5 A9 60 2F E3 EC 34 4E 8D 9B 6B F9 02 B8 fc..`/..4N..k... 00C0: 23 D3 EF B6 8A DD 76 0C 5E F6 C3 C1 DC 41 65 2A #.....v.^....Ae* 00D0: BE CC 85 09 BC 41 D4 79 27 8D 3C 23 32 4C 6F 44 .....A.y'.<#2LoD 00E0: 62 5B F4 41 39 FE 4B 47 8A FF 9F AA FB A1 BB F9 b[.A9.KG........ 00F0: 27 AE 72 C0 6A B6 78 EA BF 50 EF F8 0C DD 46 2B '.r.j.x..P....F+ ] BUILD SUCCESSFUL (total time: 0 seconds)
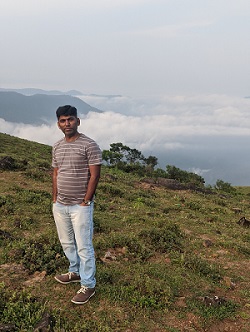
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.