Hibernate Sample Program
Table of Contents
In this post, I am going to explaining hibernate sample program and what type of files are required to create hibernate sample program.
Different types of files are required to develop the hibernate sample program.
They are
- Hibernate Jars
- Hibernate Configuration file
- Hibernate Mapping file
- POJO Class
- Client Application
1) Hibernate Jars
Before going to compile the source code we need to place the following jar files in the class path
- hibernate4.jar
- cglib-2.1.4.jar
- antlr-2.7.6.jar
- asm.jar
- jta.jar
- commons-logging-1.0.4.jar
- commons-collections-2.4.1.jar
- dom4j-1.6.1.jar
- ojdbc14.jar
2) Hibernate Configuration File (hibernate.cfg.xml)
It is an xml file used to provide the database information. Which is used to create the database connection and it will be changed database to database. In the client application we can use this xml file to get the database connection and client application by default it looks for hibernate.cfg.xml.
Here we are using MySql database.
Sample xml file ( hibernate.cfg.xml)
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernatetutorial?zeroDateTimeBehavior=convertToNull</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.show_sql">true</property> <property name="hibernate.hbm2ddl.auto">update</property> <mapping resource="student.hbm.xml"/> </session-factory> </hibernate-configuration>
Description of hibernate.cfg.xml
2.1) hibernate.dialect
By using Dialect property we can configure the appropriate database. These value will be changed database to database.
Oracle 9i/10g org.hibernate.dialect.Oracle9Dialect
Oracle any version org.hibernate.dialect.OracleDialect
MySQL org.hibernate.dialect.MySQLDialect
2.2) hibernate.hbm2ddl.auto
It allows create, update or create-drop.
2.2.1) Create
It always create new table in the database for each and every client. If the table is available (already) in the database then it will drops the table and it creates new table.
2.2.2) Update
It creates the table when the table is not available in the database. It updates the values when the table is already available. The most recommended value in all situations is Update. It is default value.
2.2.3) create-drop
It creates the table when application server is started. It drops the table when server is showdown.
Conclusion The most recommended value in all situation is update
Note
In the hibernate mapping file we can provide the mapping between java class name with table name, instance variables with table columns. If you will not configure table name and table column names in the mapping file then hibernate software creates the table with table name is pojo class name and table column names are instance variables.
2.3) hibernate.show_sql
IF show_sql=true, means that hibernate software converts the HQL queries into database specific sql queries. These queries will be displayed on the console. If shoq_sql=false, means that gibernate software generated sql queries are not displayed on the console.
2.4) hibernate.connection.pool_size
By using this property we can configure the connection pool size. The default value is 20.
3) Hibernate Mapping file
It is hear xml file of the application. In this xml file we can provides object relational mapping like calls name with table name, instance variable ( pojo class member variables) with column names.Here there is no default file name exist unlike hibernate configuration file as above. So we can give any-name.xml file acts as hibernate mapping file and we can specify this xml file in the hibernate-configuration file by using <mapping> tag.
In the hibernate application, we can develop any number of mapping files.
Sample Mapping file (student.hbm.xml)
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="com.narayanatutorial.hibernate.Student" table="student"> <id name="hid" column="HID" type="integer"> <generator class="increment"></generator> </id> <property name="sfirstname" column="SFIRSTNAME" type="string"></property> <property name="slastname" column="SLASTNAME" type="string"></property> <property name="srollnumber" column="SROLLNUMBER" type="integer"></property> </class> </hibernate-mapping>
4) POJO class
It is ordinary java bean class whose object represent table rows in the database.
Sample POJO class (StudentBean.java)
package com.narayanatutorial.hibernate; public class Student { int hid; String sfirstname; String slastname; int srollnumber; public int getSrollnumber() { return srollnumber; } public void setSrollnumber(int srollnumber) { this.srollnumber = srollnumber; } public int getHid() { return hid; } public void setHid(int hid) { this.hid = hid; } public String getSfirstname() { return sfirstname; } public void setSfirstname(String sfirstname) { this.sfirstname = sfirstname; } public String getSlastname() { return slastname; } public void setSlastname(String slastname) { this.slastname = slastname; } }
5) Client Application
Any java, j2ee application acts as hibernate application. Hibernate client application use hibernate API to activate the hibernate setup and by using pojo class and hql we can develop the database independent persistent logic.
package com.narayanatutorial.hibernate; import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class StudentApp { public static void main(String args[]) { try { String sql = ""; Configuration configuration = new Configuration().configure(); SessionFactory sf = configuration.buildSessionFactory(); Session session = sf.openSession(); Transaction tx = session.beginTransaction(); Student st = new Student(); st.setSfirstname("narayana"); st.setSlastname("ragi"); st.setSrollnumber(12345454); session.save(st); sql = "from Student"; Query query = session.createQuery(sql); List<Student> list = query.list(); for (Student st1 : list) { System.out.println("hibernate ID:"+st1.getHid()); System.out.println("Studenta First Name:"+st1.getSfirstname()); System.out.println("Student Last Name"+st1.getSlastname()); System.out.println("Student Roll Number"+st1.getSlastname()); } System.out.println("size:" + list.size()); tx.commit(); session.close(); sf.close(); } catch (Exception e) { e.printStackTrace(); } } }
Output
hibernate ID:1 Studenta First Name:Rama Student Last Namekrishna Student Roll Numberkrishna hibernate ID:2 Studenta First Name:narayana Student Last Nameswamy Student Roll Numberswamy hibernate ID:3 Studenta First Name:raju Student Last Nameswamy Student Roll Numberswamy hibernate ID:4 Studenta First Name:narayana Student Last Nameragi Student Roll Numberragi hibernate ID:5 Studenta First Name:narayana Student Last Nameragi Student Roll Numberragi size:5
Directory Structure
Description of client application class
5.1) Configuration class
It is defined in org.hibernate.cfg package. By using configuration class we can create session factory object. Configuration object encapsulate the hibernate configuration details such as connection properties, dialect and we can configure mapping details. By using this class we can load configuration file and mapping file by using configure() method. By using the following code we can get the configuration object.
Configuration configuration = new Configuration().configure();
5.2) SessionFactory
It is defined in org.hibernate package. Sessionfactory acts as the group of session objects for particular database.
Sessionfactory objects are immutable objects. Entire application having only one sessionfactory object. By using the following code we can get the sessionfactory object
Configuration configuration = new Configuration().configure(); SessionFactory sf = configuration.buildSessionFactory();
5.3) session
The main central interface between java application and database. By using sessionfactory object we get the session object.
It is recommended to use seperate session object for each client request. By using the following code we will can get the session object.
Session session = sf.openSession();
session interface contains multiple methods, here I am going to sharing some of the frequently using methods.
1) session.save(Object obj),
Here obj is persistent class object. This method is used to persistence object into database.
2) session.update(Object obj)
Here obj is persistent class object. This method is used to update persistence object into database.
3) session.saveOrUpdate(Object obj)
Here obj is persistent class object. This method is used to update or save persistence object into database.
4) session.load(Object obj, Serializable id)
Here obj is pojo class and id is hinernate generated unique id. By using this method we can abstract the object from the database.
I hope you understood the sample basic program,
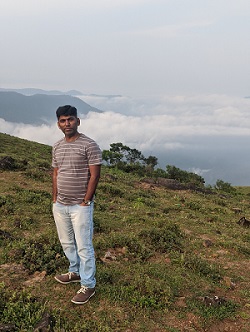
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.
good post really it works