One of the IT Companies Java Interview Questions and Answers-1
Table of Contents
1) How many objects will be created for the following scenario?
- Integer a1=10;
- Integer a1=10;
- Integer a1=20;
- Integer a1=15;
Ans: 3 objects will be created.
Explanation: Java storing value in the cache and while initialization will check in the cache, if it is exist then it will return same reference else it will create new reference and will return.
2) What is output of the following program?
package com.narayanatutorial.exaceptions; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; public class Example { public static void main(String args[]){ try{ FileInputStream fis=new FileInputStream("D:/samplefile.txt"); if(fis.read() != -1){ System.out.println((char)fis.read()); } }catch(IOException io){ System.out.println("IO Exception"); }catch(FileNotFoundException fnf){ System.out.println("FileNotFoundException Exception"); } } }
Ans: Compile error i.e exception java.io.FileNotFoundException has already been caught
Explanation: FileNotFoundException is subclass of IOException. While handling exception we need to handle subclass exception followed by super class exceptions. It means that we have to write exception blocks according to hierarchy.
3) What is the output of the following program?
package com.narayanatutorial.exaceptions; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; public class Example2 { public static void main(String args[]){ try{ int a=10; int b=a/2; }catch(IOException io){ System.out.println("IO Exception"); } } }
Ans: Compile error i.e exception java.io.IOException is never thrown in body of corresponding try statement
Explanation: Compiler will check try block is there any input or output resource utilization logic. This type of scenario applicable for checked exceptions not for the unchecked exceptions.
Example:
package com.narayanatutorial.exaceptions; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; public class Example2 { public static void main(String args[]) { try { int a = 10; int b = a / 2; } catch (NullPointerException np) { System.out.println("NullPointerException :" + np.getMessage()); } } }
4) How to swap two integers without third variable using?
package com.narayanatutorial.general;
public class SwapTwoNumbers {
public static void main(String args[]){
int a=10;
int b=20;
System.out.println("Before Swap");
System.out.println("a:"+a + " b:"+b);
a=a+b;
b=a-b;
a=a-b;
System.out.println("After Swap");
System.out.println("a:"+a + " b:"+b);
}
}
output
Before Swap
a:10 b:20
After Swap
a:20 b:10
5) How to swap two integers with third variable using?
package com.narayanatutorial.general; public class SwapTwoNumbers { public static void main(String args[]) { /* Swap two number with using third variable */ int a = 10; int b = 20; int temp = 0; System.out.println("Before Swap"); System.out.println("a:" + a + " b:" + b); temp = a; a = b; b = temp; System.out.println("After Swap"); System.out.println("a:" + a + " b:" + b); } }
6) What is difference between sendREdirect() and requestDispatcher() in jsp and servlet?
- sendRedirect takes the request from the client browser and create a new request and send back to client browser it means that it will call other application servlet. But requestDispatcher takes the request from the client browser and pay the request to other servlet withing the application
- sendRedirect will lost the previous request and requestDispatcher will not lost the previous request and it pay the copy of request to other servlet within the application
- sendRedirect pass the parameters cia session and requestDispatcher pass the parameters via request.setAttribute(param1) method
- sendRedirect request is non-transient because request URL is visible in the browser but requestDispatcher request is transient because request URL is not visible
- sendRedirect supports both i.e 1. within application 2. outside application but requestDispatcher supports within application.
- sendRedirect formance is less as compare to requestDispatcher because sendRedirect creating a new request and passing to browser.
7) How to make String s=”hello world” as reverse string programmatically?
package com.narayanatutorial.general;
public class StringReverse {
public static void main(String arg[]) {
String str = "hello world";
int len = str.length();
String reverseStr = "";
System.out.println("Original String: "+str);
for (int i = (len - 1); i >= 0; i--) {
reverseStr = reverseStr + str.charAt(i);
}
System.out.println("Reverse String: "+reverseStr);
}
}
Output
Original String: hello world
Reverse String: dlrow olleh
By using StringBuffer & StringBuilder
package com.narayanatutorial.general;
public class StringReverse {
public static void main(String arg[]) {
String str = "hello world";
System.out.println("Original String: "+str);
StringBuffer sb=new StringBuffer(str);
//StringBuilder sb=new StringBuilder(str);
String reverseStr=sb.reverse().toString();
System.out.println("Reverse String: "+reverseStr);
}
}
Output
Original String: hello world
Reverse String: dlrow olleh
8) How to sort int{1,5,1,10,3} array?
Array declared as local variable — Method Level Declaration
int[ ] a=new int[5];
a reference will be stored in the heap memory and 5 objects reference will be stored in the stack with default values 0,0,0,0,0. So total 6 objects are created.
int[ ] a;
System.out.println(“a:”+a);
we will get compiler error because local variable should be initialized before going to use.
Array declared as instance variable — Class Level Declaration
Array default value is null so it will throws NullPointerException while accessing the array object
Sorting Array Program
package com.narayanatutorial.sorting; import java.util.Arrays; public class IntegerArraySorting { public void get(){ int[] c;//=new int[3]; //If it will be enabled we will not get compiler error //System.out.println("c:"+c); //if it will be enabled we will get compiler error } public static void main(String args[]){ int[] a={2,5,1,10,3}; int[] b=new int[5]; System.out.println("before sort ascending order integer array"); for(int a1:a){ System.out.println(a1); } Arrays.sort(a); System.out.println("after sort ascending order integer array"); for(int a1:a){ System.out.println(a1); } System.out.println("---------Descending order------------"); for(int i=(a.length-1),j=0;j<a.length;i--,j++){ System.out.println("i:"+i+" j:"+j+ " a[i]:"+a[i]); b[j]=a[i]; } System.out.println("after sort descending order integer array"); for(int a1:b){ System.out.println(a1); } } } Output before sort ascending order integer array 2 5 1 10 3 after sort ascending order integer array 1 2 3 5 10 ---------Descending order------------ i:4 j:0 a[i]:10 i:3 j:1 a[i]:5 i:2 j:2 a[i]:3 i:1 j:3 a[i]:2 i:0 j:4 a[i]:1 after sort descending order integer array 10 5 3 2 1
9) What are the ways to declare Two and Three dimensional Array?
Two dimensional
- int[ ][ ] a;
- int[ ] a[ ];
- int a[ ][ ];
- int [ ]a,b[ ];
- int [ ] a, b[ ];
- int [ ] [ ]a,b;
int [ ]a,[ ]b this is the incorrect way.
Array [ ] allow only in front of the first variable not the second variable;
Three dimensional
- int [ ] [ ] [ ] a;
- int [ ] a,b[ ] ,c[ ] ;
- int [ ] [ ] [ ] a,b,c;
- int [ ] [ ] ,b,c [ ]
10) What is difference between Array and ArrayList?
Array
- Fixed size
- Not recommended in case of memory
- No methods are available to handle the data
- Performance point of view Arrays are recommended
- Hold homogeneous elements
- No ready made methods are available
- Underlying data structure is not available
- Arrays can hold primitive and objects
- Arrays can be converted into ArrayList
ArrayList
- Grow able size;
- Recommended in case of memory
- Methods are available to handle data
- Performance point of view ArrayList is not recommended
- Hold heterogeneous elements
- Ready made methods are available
- Underlying data structure is available i.e. Array
- ArrayList can hold object only not primitive data types
- ArrayList can not be converted into Array
11) What is jsp lifecycle?
- Traslation –> Converting into servlet
- Class Loader–> Loading into memory
- Instantiation –> create reference of servlet
- Initialization –>_jspInit() –> create object
- Service –> _jspService() –> service
- Destroy –> _jspDestroy –> destroying object
12) What type of comments we can use in the jsp?
- Scriptlet comments –> <%– –%>
- Html comments –><!– –>
13) What is difference between HashMap and ConcurrentHashMap?
- HashMap is not synchronized but ConcurrentHashMap is synchronized
- HashMap will give better performance compare to ConcurrentHashMap.
- HashMap follow Fail-Fast and ConcurrentHashMap will follow Safe-Fast
- HashMap will throw concurrent modification exception and ConcurrentHashMap will not that exception
- We can make HashMap as synchronized by using this method Collections.synchronizedMap(Map obj) but ConcurrentHashMap synchronized by default
- In synchronized HashMap, all methods are synchronized but in ConcurrentHashMap part of Map block are synchronized for the better performance.
- HashMap will allow one null key and multiple null values but ConcurrentHashMap will not allow null keys and null values
After synchronization of HashMap is equal-ant to HashTable
14) What is difference between Fail-Fast and Safe-Fast?
Fail-Fast
Any collection object is being iterated by one thread at the same time the same collection object is being modified by another thread then iteration will throw the concurrent modification exception. It means iteration process will be skipped at which position got exception. For example collection object having 100 objects we got exception at 20th object then remaining iteration will be skipped.
Example:
ArrayList, Vector, HashSet, HashMap and LinkedList
Key Points
- We can track the thread safety like multiple thread access the same object
- While updating the collection, data structure will be changed
Safe-Fast
Any collection object is being iterated by one thread at the same time the same collection object is being modified by another thread then iteration will not throw the concurrent modification exception. It means iteration process will be continuing until finish the iteration. For example collection object having 100 objects at 20th object collection object has been modified by another thread, it will not throw any exception and will finish complete iteration.
Example
ConcurrentHashMap
15) What is difference between throw and throw ?
Throws
- Throws will say to JVM like we are handling the exception for the method
- Syntax is throws FileNotFoundException at the end of the method declaration. For example
-
public void getData1() throws FileNotFoundException{ //To Do something }
- No need to use new operator in front of the exception class
- We can handle checked and unchecked exceptions by using throws keyword
Throw
- Throw will say to JVM like we are not handling exception and passing to you.
- Syntax is throw new NullPointerException() inside the method. For Example
-
public void getData2(){ //To Do something throw new NullPointerException(); }
- We need to use new operator to throw the exception.
- We can handle only unchecked exception by using the throw keyword
16) What is difference between String, StringBuilder, StringBuffer?
Mutable & Immutable
- String is immutable class (once created can not be changed)
- StringBuilder is mutable class (Once created we can change the value)
- StringBuffer is mutable class (Once created we can change the value)
Final & Synchronized
- String is final class and synchronized
- StringBuilder is not final class and synchronized
- StringBuffer is not final class and not synchronized
Thread Safety
- String is thread safe i.e every immutable object in java is thread safe (String can not be used by two thread simultaneously)
- StringBuffer is thread safe i.e every synchronized object in java is thread safe (StringBuffer can not be used by two thread simultaneously)
- StringBuilder is not thread safe because it is neither immutable not synchronized (StringBuilder can be used by two thread simultaneously)
Performance
- String and StringBuilder will give better performance compare to StringBuffer because of synchronization
Key Points
- StringBuffer and StringBuilder both are same excluding synchronization
- For multithread, we can go for StringBuffer and for single thread we can go for StringBuilder.
17) What is String constant pool?
String constant pool is a special type memory to store string objects. In the sting constant pool, before going to store string object, it will check whether already string object is exist or not. If exist it will not create new object and the reference point to that exist object. it not exist it will create new object with new reference.
Example
String s1=”Hello” –> String constant pool
Sting s2=”Hello” –> String constant pool
Sting s3=”Hello” –> String constant pool
Here 3 reference and value stored in the constant pool. It means only one object will be created with 3 reference in constant pool
String s=”hello”; –> String constant pool
s=”hi”; –> String constant pool
s=”bujji”; –> String constant pool
Here 1 reference and 3 values exist in the constant pool but active value is only one object that is bujji and hello, hi will be garbage so that only one object with one reference exist in the constant pool.
String s1=”hello”; –> String constant pool
String s2=new String(“hello) –> Heap memory
String s3=new String(“hello) –> Heap memory
Here 1 reference and 1 object in the constant pool and 2 objects in the hep memory. So total 3 objects are created.
String s=”hello” –> String constant pool
StringBuffer s1=new StringBuffer(“hello”); –> Heap memory
s1.append(“hi”);
String s2=”abc”; –> String constant pool
Here 2 reference and 2 objects in constant pool and 1 object in the heap memory.
String s=”hello”; –> String constant pool
String s1=”hi”; –> String constant pool
s1.concat(s); –> Heap memory
System.out.println(s);
output: hello
System.out.println(s1);
output: hi
All string method activity will be stored in the heap memory and assigned data to string will be stored in the constant pool. so that here 2 objects( hello, hi) with 2 reference in the constant pool and 1 object (hellohi) in the heap memory which will be garbaged because of no reference exist.
18) What are the steps to create user defined immutable class?
- Class should be final
- Member variables should be private
- Should avoid member variables initialization through setter() method. It means that we should initialize member variables through constructor only.
19) What are the methods are required to create user defined key for hashmap?
Method 1: hashcode method
Syntax: public native int hashcode()
Method 2: equal method
Syntax: public boolean equal(Object obj)
Note: And make that user defined class should be immutable
hashcode
This method actually exist in the object class ( super class in java)). So we are overriding that in the user defined class. By using this method we can implement unique key generation.
equal
This method actually exist in the object class. So we are overriding that method. By using this method we can check the duplicate or not.
20) What is difference between List, Map and Set?
Duplicate
- List allow duplicate objects because it is a index based storing objects
- Map will not allow duplicate keys but allow duplicate values
- Set will not allow duplicate objects
Order
- List follow the order
- Map will not follow order
- Set will not follow order
NULL
- List allow null as string number of times means duplicate allow
- Map allow one null key only , duplicate null keys are not allowed but duplicate null values are allowed
- Set will not allow null objects. It will throw NullPointerException if you try to add.
Implementation Classes
- List ==> ArrayList, Vector, LinkedList
- Map ==> HashMap, SortedMap, TreeMap
- Set ==> HashSet, SortedSet, TreeSet
Synchronization
- ArrayList, HashMap and HashSet are non-synchronized
- Vector, LinkedList, HashTable are synchronized
Performance
Deletion
LinkedList > ArrayList > Vector
Update
LinkedList > ArrayList > Vector
Insert
LinkedList > ArrayList > Vector
Iteration
ArrayList > LinkedList > Vector
General Performance
- ArrayList > VEctor
- HashMap > HashTable
- HashSet > HashTable
Miscellaneous
- SortedMap and SortedSet are follow natural order
- TreeMap and TreeSet are implementing comparable and comparator interface.
21) Can we declare variables in the interface? And Why method are static and final by default?
- We can not declare variables but we can initialize directly with public static final int 1=12;
- All methods and variables are public static final by default because interface does not have own object to access these members so that for accessing those methods and variables, we will use interface reference directly like Interface a = new <implementation class>();
Miscellaneous
We should implement all methods of interface in our implementation class other wise will give compiler exception.
22) What is bucket in HashMap?
A bucket is used to store key-value pair. Both key and value is stored in the bucket as a form of Entry object. A bucket can have multiple key-value pairs. In hashmap bucket issuing simple linked list to store objects.
23) What is performance between put() and get() methods in the HashMap?
The HashMap implementation provide constant performance for both methods i.e. both methods will give same performance.
24) How will you measure the performance of the HashMap?
An instance of the HashMap has two parameters will effect the performance of HashMap i.e. they are initial capacity and load factor
Initial Capacity
- Initial capacity is nothing the number of buckets in the hashtable.
- Initial capacity is simply the capacity at the time the hash table is created.
Load Factor
- Whenever the hashmap fulled with objects reached its initial capacity then the load factor is measure how to increase the hashmap size with initial capacity.
- When the number of entries in the hash table exceeds the product of the load factor and the current capacity, the hash table is rehashed (that is,internal data structures are rebuilt)
so that the hash table has approximately twice the number of
buckets.
25) What is default load factor of the HashMap?
0.75
26) What is design patter in the struts2.x and explain?
Struts 2.x design pattern is Pull-MVC
Struts 1.x design pattern is Push-MVC
Pull-MVC
Data model values(Parameters values) rendered from the control class.
Push-MVC
Data model values(parameters values) rendered through the request or session from the parameters
27) Struts 2.x Specialities
- Struts 2.x introduced interceptors which are already ready made code and re-usable code for the operational.
- Interceptors are not thread safe.
- Interceptors are configurable for the controller class to execute and also particular interceptors will be executed unlike filters.
- We have two stack i.e 1. basicInterceptorsStack 2.defaultInterceptorStack
- User defined interceptors also we can create and we can call particular method by using <exclude methods=””/> and <include methods=””/>
- Struts2.x is filter based controller design pattern i.e. front controller is filterDispatcherServlet. Which need to be configure in the web.xml. When application is deployed, then this filter will be initiated it means ready to use or accept requests.
- To initiate the filter no need to configure the <load-on-start> 1 </load-on-start>
- Struts2.x decreased the burden on the developers.
28) How to achieve encapsulation in java?
- Declare variable are private
- Initialize the variables through constructor
- Avoid the setter methods to set the variables values.
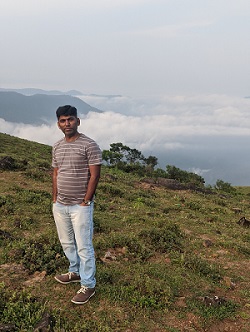
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.