Different ways to specify hibernate configuration property values
Table of Contents
In this post I am going to explaining what are the different ways to specify hibernate configuration property values.
In the hibernate there are three ways to load the hibernate property values.
- By using xml file (hibernate.config.xml)
- By using setProperty() method of configuration
- By using properties file
Hibernate mapping files are common for the above mentioned different types of hibernate configuration. In our example student.hbm.xml is a hibernate mapping file and assume this file saved in this location D:\HostingProjects\HibernateTest\build\classes\student.hbm.xml
student.hbm.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="com.narayanatutorial.hibernate.Student" table="student"> <id name="hid" column="HID" type="integer"> <generator class="increment"></generator> </id> <property name="sfirstname" column="SFIRSTNAME" type="string"></property> <property name="slastname" column="SLASTNAME" type="string"></property> <property name="srollnumber" column="SROLLNUMBER" type="integer"></property> </class> </hibernate-mapping>
1) By using xml file (hibernate.config.xml)
This is best and default hibernate configuration file to configure hibernate properties.
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernatetutorial?zeroDateTimeBehavior=convertToNull</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.show_sql">true</property> <property name="hibernate.connection.pool_size">5</property> <property name="hibernate.hbm2ddl.auto">update</property> <mapping resource="student.hbm.xml"/> </session-factory> </hibernate-configuration>
StudentApp.java
package com.narayanatutorial.hibernate; import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class StudentApp { public static void main(String args[]) { try { String sql = ""; Configuration configuration = new Configuration().configure(); SessionFactory sf = configuration.buildSessionFactory(); Session session = sf.openSession(); Transaction tx = session.beginTransaction(); Student st = new Student(); st.setSfirstname("narayana"); st.setSlastname("ragi"); st.setSrollnumber(12345454); session.save(st); sql = "from Student"; Query query = session.createQuery(sql); List<Student> list = query.list(); for (Student st1 : list) { System.out.println("hibernate ID:"+st1.getHid()); System.out.println("Studenta First Name:"+st1.getSfirstname()); System.out.println("Student Last Name"+st1.getSlastname()); System.out.println("Student Roll Number"+st1.getSlastname()); } System.out.println("size:" + list.size()); tx.commit(); session.close(); sf.close(); } catch (Exception e) { e.printStackTrace(); } } }
2) By using setProperty() method of configuration
In this we are placing hibernate configuration details like database connection and hibernate mapping file in the client application. And we need to remove the configure() method in the configuration object as follows.
StudentAppProps.java
package com.narayanatutorial.hibernate; import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class StudentAppProps { public static void main(String args[]) { try { String sql = ""; Configuration configuration = new Configuration(); configuration.setProperty("hibernate.connection.driver_class", "com.mysql.jdbc.Driver"); configuration.setProperty("hibernate.connection.url", "jdbc:mysql://localhost:3306/hibernatetutorial?zeroDateTimeBehavior=convertToNull"); configuration.setProperty("hibernate.connection.username", "root"); configuration.setProperty("hibernate.show_sql", "ture"); configuration.setProperty("hibernate.connection.pool_size", "5"); configuration.setProperty("hibernate.hbm2ddl.auto", "update"); configuration.addFile("D:\\HostingProjects\\HibernateTest\\build\\classes\\student.hbm.xml"); SessionFactory sf = configuration.buildSessionFactory(); Session session = sf.openSession(); Transaction tx = session.beginTransaction(); Student st = new Student(); st.setSfirstname("narayana"); st.setSlastname("ragi"); st.setSrollnumber(12345454); session.save(st); sql = "from Student"; Query query = session.createQuery(sql); List<Student> list = query.list(); for (Student st1 : list) { System.out.println("hibernate ID:"+st1.getHid()); System.out.println("Studenta First Name:"+st1.getSfirstname()); System.out.println("Student Last Name"+st1.getSlastname()); System.out.println("Student Roll Number"+st1.getSlastname()); } System.out.println("size:" + list.size()); tx.commit(); session.close(); sf.close(); } catch (Exception e) { e.printStackTrace(); } } }
Here we are placing hard code details directly in the client application. So this approach is not recommended.
3) By using properties file
Here we will create on property file like hibernate.properties in which we have to configure hibernate details as follows.
hibernate,properties file
It is saved in this location D:\HostingProjects\HibernateTest\build\classes\hibernate.properties
hibernate.dialect = org.hibernate.dialect.MySQLDialect hibernate.connection.driver_class = com.mysql.jdbc.Driver hibernate.connection.url = jdbc:mysql://localhost:3306/hibernatetutorial?zeroDateTimeBehavior=convertToNull hibernate.connection.username = root hibernate.show_sql = true hibernate.connection.pool_size = 5 hibernate.hbm2ddl.auto = update
And create client application like StudentAppFile.java as follows
StudentAppFile.java
package com.narayanatutorial.hibernate; import java.io.FileInputStream; import java.util.List; import java.util.Properties; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class StudentAppFile { public static void main(String args[]) { try { String sql = ""; FileInputStream fis = new FileInputStream("D:\\HostingProjects\\HibernateTest\\build\\classes\\hibernate.properties"); Properties props = new Properties(); props.load(fis); Configuration configuration = new Configuration(); configuration.setProperties(props); configuration.addFile("D:\\HostingProjects\\HibernateTest\\build\\classes\\student.hbm.xml"); SessionFactory sf = configuration.buildSessionFactory(); Session session = sf.openSession(); Transaction tx = session.beginTransaction(); Student st = new Student(); st.setSfirstname("narayana"); st.setSlastname("ragi"); st.setSrollnumber(12345454); session.save(st); sql = "from Student"; Query query = session.createQuery(sql); List<Student> list = query.list(); for (Student st1 : list) { System.out.println("hibernate ID:" + st1.getHid()); System.out.println("Studenta First Name:" + st1.getSfirstname()); System.out.println("Student Last Name" + st1.getSlastname()); System.out.println("Student Roll Number" + st1.getSlastname()); } System.out.println("size:" + list.size()); tx.commit(); session.close(); sf.close(); fis.close(); props.clear(); } catch (Exception e) { e.printStackTrace(); } } }
in this way we are loading property file by using fileinputstream instead of using hibernate API.
So we recommend you to follow first way to configure hibernate properties.
I hope you understood all these ways to specify hibernate configuration property values.
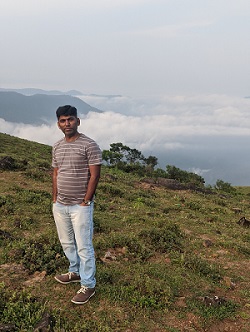
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.