Blind SQL INJECTION occurs when data input by a user is interpreted as an SQL command rather than as normal data by the backend database.
This is common vulnerability and the vulnerability by executing a test SQL query on the backend database.
Type of SQL Injections
- SQL Injections
- Blind Sql Injection
- Boolean Based Sql Injection
- Out of Band Sql Injections
It will impact on the database sensitive data by performing the following operations.
1. Reading, Updating and Deleting data or tables from the database
2. Executing the commands on the underlying operating system.
Solution :
Solution is very simple, in your project replace dynamic sql queries with parameterized sql queries.
For Example: in java, supposed you have statement which is accepting dyanmic sql queries. So you can remove it and use PreparedStatement which is accepting both dynamic and parameterized but you have to make query as parameterized as follows.
Dynamic Parameterized SQL Query Example
package DBConnection; import java.sql.Connection; import java.sql.DriverManager; import java.sql.Statement; public class CreateStatementExample { static String driver = "oracle.jdbc.driver.OracleDriver"; static String userName = ""; static String password = ""; static String url = ""; static Connection con = null; public void dataToDB(String name, int id) { Statement stmt = null; try { String SQL = "INSERT INTO XYZ(NAME, ID) VALUES('" + name + "'," + id + ")"; /* * runtime sql query generated as follows String SQL="INSERT INTO XYZ(NAME, ID) VALUES('NarayanaTutorial',123)"; */ Class.forName(driver); con = DriverManager.getConnection(url, userName, password); stmt = con.createStatement(); stmt.execute(SQL); } catch (Exception e) { e.printStackTrace(); } finally { try { if (stmt != null) { stmt.close(); } if (con != null) { con.close(); } } catch (Exception e) { } } } public static void main(String args[]) { CreateStatementExample obj = new CreateStatementExample(); obj.dataToDB("NarayanaTutorial", 123456); } }
Parameterized SQL Query Example
package DBConnection; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.Statement; public class PreparedStatementExample { static String driver = "oracle.jdbc.driver.OracleDriver"; static String userName = ""; static String password = ""; static String url = ""; static Connection con = null; public void dataToDB(String name, int id) { PreparedStatement pstmt = null; try { String SQL = "INSERT INTO XYZ(NAME, ID) VALUES(?,?)"; Class.forName(driver); con = DriverManager.getConnection(url, userName, password); pstmt = con.prepareStatement(SQL); pstmt.setString(1, name); pstmt.setInt(2, id); pstmt.execute(); } catch (Exception e) { e.printStackTrace(); } finally { try { if (pstmt != null) { pstmt.close(); } if (con != null) { con.close(); } } catch (Exception e) { } } } public static void main(String args[]){ PreparedStatementExample obj=new PreparedStatementExample(); obj.dataToDB("NarayanaTutorial", 123456); } }
I hope you understood. It is always better to use PreparedStatement parameterized to avoid all types of sql injections like Blind SQL Injection, Boolean SQL Injection, Out of Band Sql Injections, SQL Injection etc….
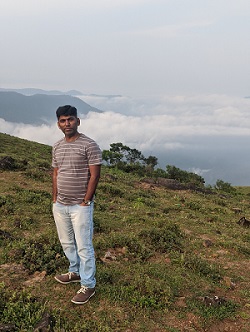
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.