In this post I am sharing most important HashMap interview questions along with answers. Java collections package is java.util, this is the most important package and used frequently in most of the projects.
What are the methods required for an object to be used as a key or value in HashMap
Table of Contents
- 1 What are the methods required for an object to be used as a key or value in HashMap
- 2 What will happen If we try to store a same key in HashMap?
- 3 Can we store a null key in HashMap?
- 4 Can we store a null key in HashMap?
- 5 Which data structure HashMap represents?
- 6 Which data structure is used to implement HashMap?
- 7 How collisions happened in HashMap?
- 8 How collisions handled in HashMap?
- 9 What will happen if more collisions happened in HashMap?
- 10 Related Posts
- equals()
- hashcode()
The key or value object should implement the above two methods. the hashcode() method is used when we insert the key object into the Map while equals() method is used when we try to retrieve a value from the same Map.
What will happen If we try to store a same key in HashMap?
If we try to store same key which is exist already in the HashMap, then it will override the old value with the new value and put() method will return the old value and there will not be any exception or error.
Can we store a null key in HashMap?
Yes.
HashMap allows one null key and which is stored at the first location of bucket array
For example: bucket[0]=value;
While storing a null key, the HashMap doesn’t call hashcode() method because it will throw NullPointerException if hashcode() method will be called.
When we call the get() method with null then the value of the first index is returned.
HashMap allow null key only once.
HashMap doesn’t allow duplicate null keys.
Can we store a null key in HashMap?
Yes.
HashMap allows null value, we can store as many null values.
Which data structure HashMap represents?
The HashMap is an implementation of HashTable data structure and which is idle for mapping one value to other value.
Which data structure is used to implement HashMap?
Even though HashMap represents a hash table, it is internally implemented by using an array and linked list data structures.
The array is used as bucket and the linked list is used to store all mapping values which land in the same bucket.
How collisions happened in HashMap?
Mulitple Keys with same hashcode.
How collisions handled in HashMap?
HashMap uses chaining to handle collisions. Which means that new entries, an object contains both key and value, are stored in a linked list along with existing value and then linked list will be stored in the bucket location.
What will happen if more collisions happened in HashMap?
Performance issue.
All the key has the same hashcode then hash table will be returned into a linked list and searching for value will take O(n) time as opposed to O(1) time.
Can we store duplicate key in HashMap?
No.
We can’t store duplicate keys in HashMap, HashMap doesn’t allow duplicate keys. If we try to insert duplicate key with same or new value then it will override the old value with new value and teh size of the HashMap remains same.
This is the reason when you get all keys from the HashMap by calling keySet() method and it returns a Set not a Collection and then Set doesn’t allow duplicates.
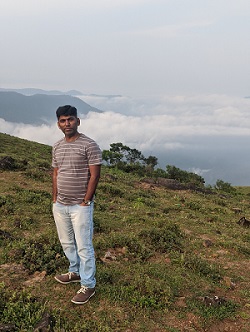
Hello! I am Narayanaswamy founder and admin of narayanatutorial.com. I have been working in the IT industry for more than 12 years. NarayanaTutorial is my web technologies blog. My specialties are Java / J2EE, Spring, Hibernate, Struts, Webservices, PHP, Oracle, MySQL, SQLServer, Web Hosting, Website Development, and IAM(ForgeRock) Specialist
I am a self-learner and passionate about training and writing. I am always trying my best to share my knowledge through my blog.